| | | Test for equal value. | | Used to test equality within a clause of a statement. | | General comparison operator. Returns -1, 0, or +1, depending on whether its receiver is less than, equal to, or greater than its argument. | , , , | Comparison operators for less than, less than or equal, greater than or equal, and greater than. | | Regular expression pattern match. | | True if the receiver and argument have both the same type and equal values. 1 == 1.0 returns , but 1.eql?(1.0) is . | | True if the receiver and argument have the same object id. | | If and Unless ExpressionsIf and unless modifiers, case expressions. when "debug" dumpDebugInfo dumpSymbols when /p\s+(\w+)/ dumpVariable($1) when "quit", "exit" exit else print "Illegal command: #{inputLine}" end | periods = Periods.new for genre in periods print genre, " " end | Break, Redo, and Next i = 0 doUntil(i > 3) { print i, " " i += 1 } | Variable Scope and LoopsRuby Programming/Syntax/Operators- 1.1 Complete List and Precedence
- 1.2 Other operators
- 1.3 Assignment
- 2.1 Local Scope
- 2.2 Global scope
- 2.3 Instance scope
- 2.4 Class scope
- 3 Default scope
- 4 Local scope gotchas
- 5 Logical And
- 6 Logical Or
- 7 References
Complete List and Precedence Operator | Name/meaning | Arguments | Precedence | Association | ! | Boolean NOT | Unary | 1 | Right | ~ | Bitwise complement | Unary | 1 | Right | + | Unary plus (no effect) | Unary | 1 | Right | ** | Exponentiation | Binary | 1 | Right | - | Unary minus (reverse sign) | Unary | 2 | Right | * | Multiplication | Binary | 3 | Left | / | Division | Binary | 3 | Left | % | Modulo (remainder) | Binary | 3 | Left | + | Addition or concatenation | Binary | 4 | Left | - | Subtraction | Binary | 4 | Left | << | Bitwise shift left or append (<< and <<- are also used in ) | Binary | 5 | Left | >> | Bitwise shift right | Binary | 5 | Left | & | Bitwise AND | Binary | 6 | Left | | | Bitwise OR | Binary | 7 | Left | ^ | Bitwise XOR | Binary | 7 | Left | < | Less-than | Binary | 8 | Left | <= | Less-than or equal-to | Binary | 8 | Left | > | Greater-than | Binary | 8 | Left | >= | Greater-than or equal to | Binary | 8 | Left | == | Equal (evaluate to the same value) | Binary | 9 | N/A | === | "Case equality", "case subsumption" or "three equals" operator. if B is a member of the set of A. Operation varies considerably depending on the data types of A and B. | Binary | 9 | N/A | != | Not equal | Binary | 9 | N/A | =~ | Pattern match | Binary | 9 | N/A | !~ | Negative pattern match | Binary | 9 | N/A | <=> | A <=> B evaluates to -1, 0, or 1; if A is less-than, equal-to, or greater-than B, respectively | Binary | 9 | N/A | && | Boolean AND | Binary | 10 | Left | || | Boolean OR | Binary | 11 | Left | .. | Range creation, boolean flip-flop | Binary | 12 | N/A | ... | Open-ended range creation, boolean flip-flop | Binary | 12 | N/A | ?: | A?B:C evaluates to B if A is true, or C if A is false | Trinary | 13 | Right | rescue | Modifier for catching exceptions e.g. | Binary | 14 | Left | = | Assignment | Binary | 15 | Right | **= | A **=B does A = A ** B | Binary | 15 | Right | *= | A *=B does A = A * B | Binary | 15 | Right | /= | A /=B does A = A / B | Binary | 15 | Right | %= | A %=B does A = A % B | Binary | 15 | Right | += | A +=B does A = A + B | Binary | 15 | Right | -= | A -=B does A = A – B | Binary | 15 | Right | <<= | A <<=B does A = A << B | Binary | 15 | Right | >>= | A >>=B does A = A >> B | Binary | 15 | Right | &&= | A &&=B assigns B to A if A is true or not nil | Binary | 15 | Right | &= | A &=B does A = A & B | Binary | 15 | Right | ||= | A ||=B assigns B to A if A is nil or false | Binary | 15 | Right | |= | A |= B does A = A | B | Binary | 15 | Right | ^= | A ^=B does A = A ^ B | Binary | 15 | Right | defined? | nil if the expression cannot be evaluated (e.g. unset variable) | Unary | 16 | N/A | not | Boolean NOT | Unary | 17 | Right | and | Boolean AND | Binary | 18 | Left | or | Boolean OR | Binary | 18 | Left | if | Conditional, e.g. | Binary | 19 | N/A | unless | Negative conditional, e.g. | Binary | 19 | N/A | while | Loop conditional, e.g. | Binary | 19 | N/A | until | Loop conditional, e.g. | Binary | 19 | N/A | Higher precedence (lower number in the above table) operators have their immediate arguments evaluated first. Precedence order can be altered with () blocks. For example, because * has higher precedence than +, then: 1 + 2 * 3 == 7 (1 + 2) * 3 == 9 Association direction controls which operators have their arguments evaluated first when multiple operators with the same precedence appear in a row. For example, because - has left association: 1 – 2 – 3 == (1 – 2) – 3 == -1 – 3 == -4 instead of: 1 – 2 – 3 == 1 – (2 – 3) == 1 - -1 == 0 Because ** has right association: 2 ** 3 ** 2 == 2 ** (3 ** 2) == 2 ** 9 == 512 2 ** 3 ** 2 == (2 ** 3) ** 2 == 8 ** 2 == 64 {} blocks have lower precedence than the above operators, followed by do/end blocks. Array accesses with [] can be thought of as having a higher precedence than any above operator. The operators ** through !~ can be overridden (defined for new classes, or redefined for existing operations). Note that rescue, if, unless, while, and until are operators when used as modifiers in one-liners (as in the above examples) but can also be used as keywords. Other operatorsThe dot operator . is used for calling methods on objects, also known as passing a message to the object. Ruby 2.3.0 introduced the safe navigation operator &. , also known as the "lonely operator". [2] This allows replacing An equivalent .dig() method was introduced for hashes and arrays: are safer versions of: The safe navigation operator will raise an error if a requested method, key, or index is not available; unlike the technique of using try() for this purpose, which will return nil. [3] Yukihiro Matsumoto considered ! ?. and .? before settling on &. because: [4] - ?. conflicts with *?
- ?. is used by other languages, thus .? is confusingly similar but different
- ! conflicts with "not" logic
- ? is already used by convention for functions that return booleans
- &. is reminiscent of the && syntax the operator is replacing
!! is sometimes seen, but this is simply the ! operator twice. It is used to force the following expression to evaluate to a boolean. This technique is considered non-idiomatic and poor programming practice, because there are more explicit ways to force such a conversion (which is rarely needed to begin with). Assignment in Ruby is done using the equal operator "=". This is both for variables and objects, but since strings, floats, and integers are actually objects in Ruby, you're always assigning objects. Self assignment A frequent question from C and C++ types is "How do you increment a variable? Where are ++ and -- operators?" In Ruby, one should use x+=1 and x-=1 to increment or decrement a variable. Multiple assignments Conditional assignment Operator ||= is a shorthand form that closely resembles the expression: [5] Operator ||= can be shorthand for code like: In same way &&= operator works: Operator &&= is a shorthand form of the expression: In Ruby there's a local scope, a global scope, an instance scope, and a class scope. Local ScopeThis error appears because this x(toplevel) is not the x(local) inside the do..end block the x(local) is a local variable to the block, whereas when trying the puts x(toplevel) we're calling a x variable that is in the top level scope, and since there's not one, Ruby protests. Global scopeThis output is given because prefixing a variable with a dollar sign makes the variable a global. Instance scopeWithin methods of a class, you can share variables by prefixing them with an @. Class scopeA class variable is one that is like a "static" variable in Java. It is shared by all instances of a class. Here's a demo showing the various types: This will print the two lines "kiwi" and "kiwi told you so!!", then fail with a undefined local variable or method 'localvar' for #<Test:0x2b36208 @instvar="kiwi"> (NameError). Why? Well, in the scope of the method print_localvar there doesn't exists localvar, it exists in method initialize(until GC kicks it out). On the other hand, class variables '@@classvar' and '@instvar' are in scope across the entire class and, in the case of @@class variables, across the children classes. Class variables have the scope of parent class AND children, these variables can live across classes, and can be affected by the children actions ;-) This new child of Test also has @@classvar with the original value newvar.print_classvar. The value of @@classvar has been changed to 'kiwi kiwi waaai!!' This shows that @@classvar is "shared" across parent and child classes. Default scopeWhen you don't enclose your code in any scope specifier, ex: it affects the default scope, which is an object called "main". For example, if you had one script that says and other_script.rb says They could share variables. Note however, that the two scripts don't share local variables. Local scope gotchasTypically when you are within a class, you can do as you'd like for definitions, like. And also, procs "bind" to their surrounding scope, like However, the "class" and "def" keywords cause an *entirely new* scope. You can get around this limitation by using define_method, which takes a block and thus keeps the outer scope (note that you can use any block you want, to, too, but here's an example). Here's using an arbitrary block Logical AndThe binary "and" operator will return the logical conjunction of its two operands. It is the same as "&&" but with a lower precedence. Example: The binary "or" operator will return the logical disjunction of its two operands. It is the same as "||" but with a lower precedence. Example: - ↑ http://ruby-doc.org/core-2.4.0/doc/syntax/precedence_rdoc.html
- ↑ https://www.ruby-lang.org/en/news/2015/12/25/ruby-2-3-0-released/
- ↑ http://blog.rubyeffect.com/ruby-2-3s-lonely-operator/
- ↑ https://bugs.ruby-lang.org/issues/11537
- ↑ http://www.rubyinside.com/what-rubys-double-pipe-or-equals-really-does-5488.html
 Navigation menuRuby 2.7 Reference SAVE UKRAINEIn Ruby, assignment uses the = (equals sign) character. This example assigns the number five to the local variable v : Assignment creates a local variable if the variable was not previously referenced. Abbreviated AssignmentYou can mix several of the operators and assignment. To add 1 to an object you can write: This is equivalent to: You can use the following operators this way: + , - , * , / , % , ** , & , | , ^ , << , >> There are also ||= and &&= . The former makes an assignment if the value was nil or false while the latter makes an assignment if the value was not nil or false . Here is an example: Note that these two operators behave more like a || a = 0 than a = a || 0 . Multiple AssignmentYou can assign multiple values on the right-hand side to multiple variables: In the following sections any place “variable” is used an assignment method, instance, class or global will also work: You can use multiple assignment to swap two values in-place: If you have more values on the right hand side of the assignment than variables on the left hand side, the extra values are ignored: You can use * to gather extra values on the right-hand side of the assignment. The * can appear anywhere on the left-hand side: But you may only use one * in an assignment. Array DecompositionLike Array decomposition in method arguments you can decompose an Array during assignment using parenthesis: You can decompose an Array as part of a larger multiple assignment: Since each decomposition is considered its own multiple assignment you can use * to gather arguments in the decomposition:  - Ruby Basics
- Ruby - Home
- Ruby - Overview
- Ruby - Environment Setup
- Ruby - Syntax
- Ruby - Classes and Objects
- Ruby - Variables
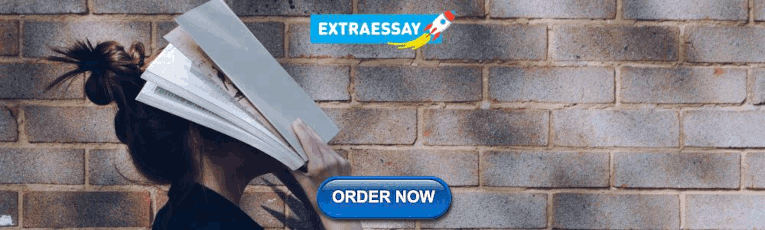 Ruby - Operators- Ruby - Comments
- Ruby - IF...ELSE
- Ruby - Loops
- Ruby - Methods
- Ruby - Blocks
- Ruby - Modules
- Ruby - Strings
- Ruby - Arrays
- Ruby - Hashes
- Ruby - Date & Time
- Ruby - Ranges
- Ruby - Iterators
- Ruby - File I/O
- Ruby - Exceptions
- Ruby Advanced
- Ruby - Object Oriented
- Ruby - Regular Expressions
- Ruby - Database Access
- Ruby - Web Applications
- Ruby - Sending Email
- Ruby - Socket Programming
- Ruby - Ruby/XML, XSLT
- Ruby - Web Services
- Ruby - Tk Guide
- Ruby - Ruby/LDAP Tutorial
- Ruby - Multithreading
- Ruby - Built-in Functions
- Ruby - Predefined Variables
- Ruby - Predefined Constants
- Ruby - Associated Tools
- Ruby Useful Resources
- Ruby - Quick Guide
- Ruby - Useful Resources
- Ruby - Discussion
- Ruby - Ruby on Rails Tutorial
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Ruby supports a rich set of operators, as you'd expect from a modern language. Most operators are actually method calls. For example, a + b is interpreted as a.+(b), where the + method in the object referred to by variable a is called with b as its argument. For each operator (+ - * / % ** & | ^ << >> && ||), there is a corresponding form of abbreviated assignment operator (+= -= etc.). Ruby Arithmetic OperatorsAssume variable a holds 10 and variable b holds 20, then − Operator | Description | Example | + | Addition − Adds values on either side of the operator. | a + b will give 30 | − | Subtraction − Subtracts right hand operand from left hand operand. | a - b will give -10 | * | Multiplication − Multiplies values on either side of the operator. | a * b will give 200 | / | Division − Divides left hand operand by right hand operand. | b / a will give 2 | % | Modulus − Divides left hand operand by right hand operand and returns remainder. | b % a will give 0 | ** | Exponent − Performs exponential (power) calculation on operators. | a**b will give 10 to the power 20 | Ruby Comparison Operators Operator | Description | Example | == | Checks if the value of two operands are equal or not, if yes then condition becomes true. | (a == b) is not true. | != | Checks if the value of two operands are equal or not, if values are not equal then condition becomes true. | (a != b) is true. | > | Checks if the value of left operand is greater than the value of right operand, if yes then condition becomes true. | (a > b) is not true. | < | Checks if the value of left operand is less than the value of right operand, if yes then condition becomes true. | (a < b) is true. | >= | Checks if the value of left operand is greater than or equal to the value of right operand, if yes then condition becomes true. | (a >= b) is not true. | <= | Checks if the value of left operand is less than or equal to the value of right operand, if yes then condition becomes true. | (a <= b) is true. | <=> | Combined comparison operator. Returns 0 if first operand equals second, 1 if first operand is greater than the second and -1 if first operand is less than the second. | (a <=> b) returns -1. | === | Used to test equality within a when clause of a statement. | (1...10) === 5 returns true. | .eql? | True if the receiver and argument have both the same type and equal values. | 1 == 1.0 returns true, but 1.eql?(1.0) is false. | equal? | True if the receiver and argument have the same object id. | if aObj is duplicate of bObj then aObj == bObj is true, a.equal?bObj is false but a.equal?aObj is true. | Ruby Assignment Operators Operator | Description | Example | = | Simple assignment operator, assigns values from right side operands to left side operand. | c = a + b will assign the value of a + b into c | += | Add AND assignment operator, adds right operand to the left operand and assign the result to left operand. | c += a is equivalent to c = c + a | -= | Subtract AND assignment operator, subtracts right operand from the left operand and assign the result to left operand. | c -= a is equivalent to c = c - a | *= | Multiply AND assignment operator, multiplies right operand with the left operand and assign the result to left operand. | c *= a is equivalent to c = c * a | /= | Divide AND assignment operator, divides left operand with the right operand and assign the result to left operand. | c /= a is equivalent to c = c / a | %= | Modulus AND assignment operator, takes modulus using two operands and assign the result to left operand. | c %= a is equivalent to c = c % a | **= | Exponent AND assignment operator, performs exponential (power) calculation on operators and assign value to the left operand. | c **= a is equivalent to c = c ** a | Ruby Parallel AssignmentRuby also supports the parallel assignment of variables. This enables multiple variables to be initialized with a single line of Ruby code. For example − This may be more quickly declared using parallel assignment − Parallel assignment is also useful for swapping the values held in two variables − Ruby Bitwise OperatorsBitwise operator works on bits and performs bit by bit operation. Assume if a = 60; and b = 13; now in binary format they will be as follows − The following Bitwise operators are supported by Ruby language. Operator | Description | Example | & | Binary AND Operator copies a bit to the result if it exists in both operands. | (a & b) will give 12, which is 0000 1100 | | | Binary OR Operator copies a bit if it exists in either operand. | (a | b) will give 61, which is 0011 1101 | ^ | Binary XOR Operator copies the bit if it is set in one operand but not both. | (a ^ b) will give 49, which is 0011 0001 | ~ | Binary Ones Complement Operator is unary and has the effect of 'flipping' bits. | (~a ) will give -61, which is 1100 0011 in 2's complement form due to a signed binary number. | << | Binary Left Shift Operator. The left operands value is moved left by the number of bits specified by the right operand. | a << 2 will give 240, which is 1111 0000 | >> | Binary Right Shift Operator. The left operands value is moved right by the number of bits specified by the right operand. | a >> 2 will give 15, which is 0000 1111 | Ruby Logical OperatorsThe following logical operators are supported by Ruby language Operator | Description | Example | and | Called Logical AND operator. If both the operands are true, then the condition becomes true. | (a and b) is true. | or | Called Logical OR Operator. If any of the two operands are non zero, then the condition becomes true. | (a or b) is true. | && | Called Logical AND operator. If both the operands are non zero, then the condition becomes true. | (a && b) is true. | || | Called Logical OR Operator. If any of the two operands are non zero, then the condition becomes true. | (a || b) is true. | ! | Called Logical NOT Operator. Use to reverses the logical state of its operand. If a condition is true, then Logical NOT operator will make false. | !(a && b) is false. | not | Called Logical NOT Operator. Use to reverses the logical state of its operand. If a condition is true, then Logical NOT operator will make false. | not(a && b) is false. | Ruby Ternary OperatorThere is one more operator called Ternary Operator. It first evaluates an expression for a true or false value and then executes one of the two given statements depending upon the result of the evaluation. The conditional operator has this syntax − Operator | Description | Example | ? : | Conditional Expression | If Condition is true ? Then value X : Otherwise value Y | Ruby Range OperatorsSequence ranges in Ruby are used to create a range of successive values - consisting of a start value, an end value, and a range of values in between. In Ruby, these sequences are created using the ".." and "..." range operators. The two-dot form creates an inclusive range, while the three-dot form creates a range that excludes the specified high value. Operator | Description | Example | .. | Creates a range from start point to end point inclusive. | 1..10 Creates a range from 1 to 10 inclusive. | ... | Creates a range from start point to end point exclusive. | 1...10 Creates a range from 1 to 9. | Ruby defined? Operatorsdefined? is a special operator that takes the form of a method call to determine whether or not the passed expression is defined. It returns a description string of the expression, or nil if the expression isn't defined. There are various usage of defined? Operator For Example Ruby Dot "." and Double Colon "::" OperatorsYou call a module method by preceding its name with the module's name and a period, and you reference a constant using the module name and two colons. The :: is a unary operator that allows: constants, instance methods and class methods defined within a class or module, to be accessed from anywhere outside the class or module. Remember in Ruby, classes and methods may be considered constants too. You need to just prefix the :: Const_name with an expression that returns the appropriate class or module object. If no prefix expression is used, the main Object class is used by default. Here are two examples − Second Example Ruby Operators PrecedenceThe following table lists all operators from highest precedence to lowest. Method | Operator | Description | Yes | :: | Constant resolution operator | Yes | [ ] [ ]= | Element reference, element set | Yes | ** | Exponentiation (raise to the power) | Yes | ! ~ + - | Not, complement, unary plus and minus (method names for the last two are +@ and -@) | Yes | * / % | Multiply, divide, and modulo | Yes | + - | Addition and subtraction | Yes | >> << | Right and left bitwise shift | Yes | & | Bitwise 'AND' | Yes | ^ | | Bitwise exclusive `OR' and regular `OR' | Yes | <= < > >= | Comparison operators | Yes | <=> == === != =~ !~ | Equality and pattern match operators (!= and !~ may not be defined as methods) | | && | Logical 'AND' | | || | Logical 'OR' | | .. ... | Range (inclusive and exclusive) | | ? : | Ternary if-then-else | | = %= { /= -= += |= &= >>= <<= *= &&= ||= **= | Assignment | | defined? | Check if specified symbol defined | | not | Logical negation | | or and | Logical composition | NOTE − Operators with a Yes in the method column are actually methods, and as such may be overridden. - Ruby Tutorial
- Ruby Operators Precedence
- Ruby Arithmetic Operators
- Ruby Comparison Operators
Ruby Assignment Operators- Ruby Parallel Assignment
- Ruby Bitwise Operators
- Ruby Logical Operators
- Ruby Ternary operator
- Ruby Defined Operators
- Ruby Dot And Double Colon Operators
Assignment OperatorsIn Ruby assignment operator is done using the equal operator "=". This is applicable both for variables and objects, as strings, floats, and integers are actually objects in Ruby, you're always assigning objects. Operator | Name | Description | Example | = | Equal operator "=" | Simple assignment operator, Assigns values from right side operands to left side operand | z = x + y will assign value of a + b into c | += | Add AND | Adds right operand to the left operand and assign the result to left operand | x += y is equivalent to x = x + y | -= | Subtract AND | Subtracts right operand from the left operand and assign the result to left operand | x -= y is equivalent to x = x - y | *= | Multiply AND | Multiplies right operand with the left operand and assign the result to left operand | x *= y is equivalent to x = x * y | /= | Divide AND | Divides left operand with the right operand and assign the result to left operand | x /= y is equivalent to x = x / y | %= | Modulus AND | Takes modulus using two operands and assign the result to left operand | x %= y is equivalent to x = x % y | **= | Exponent AND | Performs exponential calculation on operators and assign value to the left operand | x **= y is equivalent to x = x ** y | Example: Ruby assignment operator Previous: Ruby Comparison Operators Next: Ruby Parallel Assignment Follow us on Facebook and Twitter for latest update. - Weekly Trends and Language Statistics
Ruby Language- Getting started with Ruby Language
- Awesome Book
- Awesome Community
- Awesome Course
- Awesome Tutorial
- Awesome YouTube
- Blocks and Procs and Lambdas
- C Extensions
- Casting (type conversion)
- Catching Exceptions with Begin / Rescue
- Command Line Apps
- Control Flow
- Design Patterns and Idioms in Ruby
- Destructuring
- Dynamic Evaluation
- Enumerable in Ruby
- Enumerators
- Environment Variables
- File and I/O Operations
- Gem Creation/Management
- Generate a random number
- Getting started with Hanami
- Implicit Receivers and Understanding Self
- Inheritance
- Installation
- instance_eval
- Introspection
- Introspection in Ruby
- JSON with Ruby
- Keyword Arguments
- Loading Source Files
- Message Passing
- Metaprogramming
- method_missing
- Monkey Patching in Ruby
- Multidimensional Arrays
- Operating System or Shell commands
- Assignment Operators
- Comparison Operators
- OptionParser
- Pure RSpec JSON API testing
- Recursion in Ruby
- Refinements
- Regular Expressions and Regex Based Operations
- Ruby Access Modifiers
- Ruby Version Manager
- Singleton Class
- Special Constants in Ruby
- Splat operator (*)
- Variable Scope and Visibility
Ruby Language Operators Assignment OperatorsFastest entity framework extensions, simple assignment. = is a simple assignment. It creates a new local variable if the variable was not previously referenced. This will output: Parallel AssignmentVariables can also be assigned in parallel, e.g. x, y = 3, 9 . This is especially useful for swapping values: Abbreviated AssignmentIt's possible to mix operators and assignment. For example: Shows the following output: Various operations can be used in abbreviated assignment: Operator | Description | Example | Equivalent to |
---|
| Adds and reassigns the variable | | | | Subtracts and reassigns the variable | | | | Multiplies and reassigns the variable | | | | Divides and reassigns the variable | | | | Divides, takes the remainder, and reassigns the variable | | | | Calculates the exponent and reassigns the variable | | |
Got any Ruby Language Question? - Advertise with us
- Cookie Policy
- Privacy Policy
Get monthly updates about new articles, cheatsheets, and tricks. Ruby Tricks, Idiomatic Ruby, Refactorings and Best PracticesConditional assignment. Ruby is heavily influenced by lisp. One piece of obvious inspiration in its design is that conditional operators return values. This makes assignment based on conditions quite clean and simple to read. There are quite a number of conditional operators you can use. Optimize for readability, maintainability, and concision. If you're just assigning based on a boolean, the ternary operator works best (this is not specific to ruby/lisp but still good and in the spirit of the following examples!). If you need to perform more complex logic based on a conditional or allow for > 2 outcomes, if / elsif / else statements work well. If you're assigning based on the value of a single variable, use case / when A good barometer is to think if you could represent the assignment in a hash. For example, in the following example you could look up the value of opinion in a hash that looks like {"ANGRY" => comfort, "MEH" => ignore ...} - Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack OverflowFind centralized, trusted content and collaborate around the technologies you use most. Q&A for work Connect and share knowledge within a single location that is structured and easy to search. Get early access and see previews of new features. How do I use the conditional operator (? :) in Ruby?How is the conditional operator ( ? : ) used in Ruby? For example, is this correct? - ternary-operator
- conditional-operator
 - 1 yes, I think, but I also think you could accomplish that by: question=question[0,20] If it was smaller than 20, it won't change it any. – DGM Commented Nov 23, 2010 at 5:06
- i also need to add a '...' if length is greater than 20 – Mithun Sreedharan Commented Nov 23, 2010 at 5:32
- 1 Be careful blindly chopping off a line at a given column. You can end up cutting a word midway then appending the elipsis ('...'), which looks bad. Instead, look for a nearby punctuation or whitespace character, and truncate there. Only if there is no better breaking point nearby should you truncate mid-word. – the Tin Man Commented Nov 23, 2010 at 6:11
7 Answers 7It is the ternary operator , and it works like in C (the parenthesis are not required). It's an expression that works like: However, in Ruby, if is also an expression so: if a then b else c end === a ? b : c , except for precedence issues. Both are expressions. Note that in the first case parenthesis are required (otherwise Ruby is confused because it thinks it is puts if 1 with some extra junk after it), but they are not required in the last case as said issue does not arise. You can use the "long-if" form for readability on multiple lines:  - Puts 0 ? 2 : 3 also gives 2 as a result. Why is that? – X_Trust Commented Jul 9, 2014 at 15:23
- 19 @X_Trust In Ruby, the only falsy values are nil and false . Not very usual, indeed. – Kroltan Commented Jul 25, 2014 at 14:04
- This is a good explanation, but like almost every explanation I've seen, the behavior is essentially described as condition ? value if true : value if false . I think a much better way to describe the behavior is this: condition ? value if TRUTHY : value if FALSEY . The difference is subtle but very important to understand. – user16452228 Commented Feb 10, 2022 at 19:12
 - Terse but explains what it does. – the Tin Man Commented Oct 2, 2014 at 23:17
- 4 Small edit puts (true ? "true" : "false") with parenthesis. Otherwise the order of operations is not clear. When I first read this I was confused as I read it as (puts true) ? "true" : "false" then expected puts to return the boolean which then became the string value. – Fresheyeball Commented Aug 25, 2015 at 23:16
Your use of ERB suggests that you are in Rails. If so, then consider truncate , a built-in helper which will do the job for you: - This is great! what I exactly want to do!! – Mithun Sreedharan Commented Nov 24, 2010 at 4:07
- 12 This is years late, but I was very impressed with this answer as it jumped past all the syntactical aspects and went right to what the questioner was trying to accomplish. – Mike Buckbee Commented May 5, 2014 at 5:21
- 2 +1, but erb does not necessarily imply rails (Sinatra, standalone ERB, etc). – tew Commented Aug 2, 2014 at 8:43
@pst gave a great answer, but I'd like to mention that in Ruby the ternary operator is written on one line to be syntactically correct, unlike Perl and C where we can write it on multiple lines: Normally Ruby will raise an error if you attempt to split it across multiple lines, but you can use the \ line-continuation symbol at the end of a line and Ruby will be happy: This is a simple example, but it can be very useful when dealing with longer lines as it keeps the code nicely laid out. It's also possible to use the ternary without the line-continuation characters by putting the operators last on the line, but I don't like or recommend it: I think that leads to really hard to read code as the conditional test and/or results get longer. I've read comments saying not to use the ternary operator because it's confusing, but that is a bad reason to not use something. By the same logic we shouldn't use regular expressions, range operators (' .. ' and the seemingly unknown "flip-flop" variation). They're powerful when used correctly, so we should learn to use them correctly. Why have you put brackets around true ? Consider the OP's example: Wrapping the conditional test helps make it more readable because it visually separates the test: Of course, the whole example could be made a lot more readable by using some judicious additions of whitespace. This is untested but you'll get the idea: Or, more written more idiomatically: It'd be easy to argument that readability suffers badly from question.question too. - 2 If multi-line, why not just use if...else...end? – Wayne Conrad Commented Nov 23, 2010 at 16:44
- 2 Because of too many years working in Perl and C? I use either, depending on the situation and whether one is visually clearer than the other. Sometimes if/else is too verbose, sometimes ?: is ugly. – the Tin Man Commented Nov 23, 2010 at 16:47
- 1 @WayneConrad The if has at least one problem explained in this answer: stackoverflow.com/a/4252945/2597260 Compare a few ways of using multiline if/ternary operator: gist.github.com/nedzadarek/0f9f99755d42bad10c30 – Darek Nędza Commented Dec 17, 2014 at 18:14
- Why have you put brackets around true ? – Zac Commented Oct 7, 2016 at 17:05
- 2 Because true is actually sitting in for what would be an expression that evaluates to true or false . It's better to visually delimit those since ternary statements can quickly devolve into visual noise, reducing readability which affects maintainability. – the Tin Man Commented Oct 7, 2016 at 17:17
A simple example where the operator checks if player's id is 1 and sets enemy id depending on the result And I found a post about to the topic which seems pretty helpful.  - 4 Why not enemy_id = player_id == 1 ? 2 : 1 ? – Aaron Blenkush Commented Mar 19, 2018 at 19:51
- 1 @AaronBlenkush Thanks for the elegant input. I am still in noob level, probably it is why :) – devwanderer Commented Mar 21, 2018 at 7:01
Easiest way: since param_a is not equal to param_b then the result 's value will be Not same!  The code condition ? statement_A : statement_B is equivalent to  Your AnswerReminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more Sign up or log inPost as a guest. Required, but never shown By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy . Not the answer you're looking for? Browse other questions tagged ruby ternary-operator conditional-operator or ask your own question .- The Overflow Blog
- Scaling systems to manage all the metadata ABOUT the data
- Navigating cities of code with Norris Numbers
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions- A study on the speed of gravity
- How do you "stealth" a relativistic superweapon?
- What is the purpose of toroidal magnetic field in tokamak fusion device?
- Why is this white line between the two vertical boxes?
- Are there jurisdictions where an uninvolved party can appeal a court decision?
- Many and Many of - a subtle difference in meaning?
- What connotation does "break out the checkbook" have?
- I submitted a paper and later realised one reference was missing, although I had written the authors in the body text. What could happen?
- What exactly is component based architecture and how do I get it to work?
- Would donations count as revenue from a free software?
- How would a culture living in an extremely vertical environment deal with dead bodies?
- How to cite a book if only its chapters have DOIs?
- Who became an oligarch after the collapse of the USSR
- Venus’ LIP period starts today, can we save the Venusians?
- Do "Whenever X becomes the target of a spell" abilities get triggered by counterspell?
- can a CPU once removed retain information that poses a security concern?
- Trying to understand an attack showcased in a viral YouTube video
- How did Jason Bourne know the garbage man isn't CIA?
- Power line crossing data lines via the ground plane
- Age is just a number!
- Terminal autocomplete (tab) not completing when changing directory up one level (cd ../)
- Sulphur smell in Hot water only
- Questions about best way to raise the handlebar on my bike
- Why was I was allowed to bring 1.5 liters of liquid through security at Frankfurt Airport?
 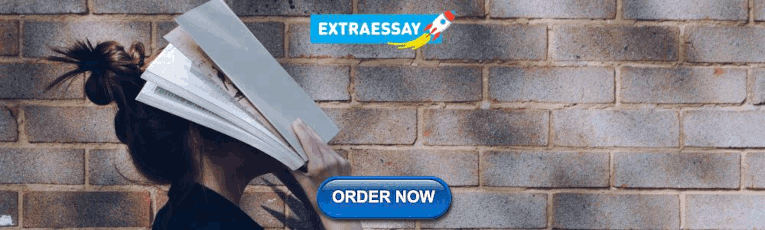 |
COMMENTS
Because += is just a shorthand for the full expression.. If it were a message of its own, then adding operator behavior for a class would require defining an assignment operator for each of the shorthand combinations, in addition to the already-probably-required operators for plain assignment and each binary operator.
Assignment methods can not be defined using the shorthand syntax. These are method names for the various Ruby operators. Each of these operators accepts only one argument. Following the operator is the typical use or name of the operator. Creating an alternate meaning for the operator may lead to confusion as the user expects plus to add things ...
by Jesus Castello. Ruby has a lot of interesting operators. Like: The spaceship operator ( <=>) The modulo assignment operator ( %=) The triple equals ( ===) operator. Greater than ( >) & less than ( <) Not equals ( !=) What you may not realize is that many of these operators are actually Ruby methods.
Assignment ¶ ↑. In Ruby, assignment uses the = (equals sign) character. This example assigns the number five to the local variable v: v = 5. Assignment creates a local variable if the variable was not previously referenced. An assignment expression result is always the assigned value, including assignment methods.
Ruby has the basic set of operators (+, -, *, /, and so on) as well as a few surprises. A complete list of the operators, and their precedences, is given in Table 18.4 on page 219. In Ruby, many operators are actually method calls. When you write a*b+c you're actually asking the object referenced by a to execute the method `` * '', passing in ...
Assignment in Ruby is done using the equal operator "=". This is both for variables and objects, but since strings, floats, and integers are actually objects in Ruby, you're always assigning objects. Examples: myvar = 'myvar is now this string' var = 321 dbconn = Mysql::new('localhost','root','password') Self assignment.
In Ruby, operators such as +, are defined as methods on the class. Literals define their methods within the lower level, C language. String class, for example. Ruby objects can define or overload their own implementation for most operators. Here is an example: self. concat ( str ). concat ( "another string" ) end end foobar = Foo. new ( "test ...
6. A silly, syntactical question: If the assignment operator is really a function, like. def value=(x) @value = x. end. without a space between the left-hand operand and the "=", then why can the assignment be made as test.value = x (with a space), but the method definition cannot be written as: def value = (x)
Assignment. In Ruby, assignment uses the = ... You can mix several of the operators and assignment. To add 1 to an object you can write: a = 1 a += 2 p a # prints 3. This is equivalent to: a = 1 a = a + 2 p a # prints 3. ... In the following sections any place "variable" is used an assignment method, instance, class or global will also work ...
1. print foo_total += f. The output of this code sample will be 10 30 60 100 150 210 210 but I'm actually looking for 10 20 30 40 50 60 210 I know that it can be achieved by using the following code. print f. foo_total += f.
Ruby - Operators - Ruby supports a rich set of operators, as you'd expect from a modern language. Most operators are actually method calls. For example, a + b is interpreted as a.+(b), where the + method in the object referred to by variable a is called with b as its argument.
What are assignment methods in ruby? The methods in which we use the suffix equals (=) are called assignment methods. The suffix indicates that we can use the method to change the state of the ...
Equal operator "=". Simple assignment operator, Assigns values from right side operands to left side operand. z = x + y will assign value of a + b into c. Add AND. Adds right operand to the left operand and assign the result to left operand. x += y is equivalent to x = x + y. Subtract AND.
For assignment methods the return value is ignored, the arguments are returned instead. These are method names for the various ruby operators. Each of these operators accept only one argument. Following the operator is the typical use or name of the operator. Creating an alternate meaning for the operator may lead to confusion as the user ...
Abbreviated Assignment. It's possible to mix operators and assignment. For example: x = 1 y = 2 puts "x is #{x}, y is #{y}" x += y puts "x is now #{x}" Shows the following output: x is 1, y is 2 x is now 3 Various operations can be used in abbreviated assignment:
Setter methods may look like assignment, but semantically they are method calls. I think it just confuses matters to call setter method invocation an 'assignment operation'. The desire to override assignment or define an assignment operator is generally indicative of some misunderstanding regarding Ruby's object model. Gary W.
Since ruby parses the bare a left of the if first and has not yet seen an assignment to a it assumes you wish to call a method. Ruby then sees the assignment to a and will assume you are referencing a local method. ... You can mix several of the operators and assignment. To add 1 to an object you can write: a = 1 a += 2 p a # prints 3. This is ...
Here is a a simplified use of the conditional assignment operator to illustrate the definition: a ||= b. If a is false, nil or undefined, then evaluate b and set a to the result. This seems to ...
Conditional assignment. Ruby is heavily influenced by lisp. One piece of obvious inspiration in its design is that conditional operators return values. This makes assignment based on conditions quite clean and simple to read. There are quite a number of conditional operators you can use. Optimize for readability, maintainability, and concision.
Ruby's use of => as the "assignment" operator for hashes comes from Perl, which uses the same operator, however, => doesn't mean "assign". Instead, think of it meaning "associated with". In Perl, => is really an alias for , which ends up defining an array of the key and value pair. So, in Ruby, don't think of it as assignment either and think of it as association again.
It is the ternary operator, and it works like in C (the parenthesis are not required). It's an expression that works like: if_this_is_a_true_value ? then_the_result_is_this : else_it_is_this However, in Ruby, if is also an expression so: if a then b else c end === a ? b : c, except for precedence issues. Both are expressions.