Learn Python practically and Get Certified .
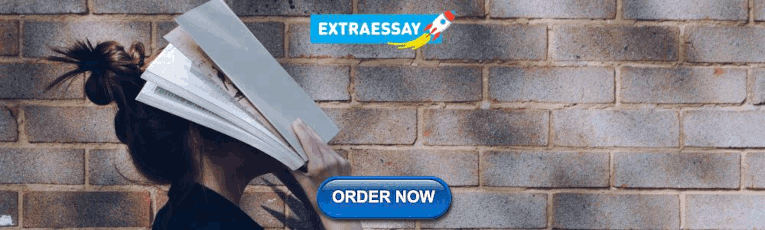
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Get Started With Python
- Your First Python Program
- Python Comments
Python Fundamentals
- Python Variables and Literals
- Python Type Conversion
- Python Basic Input and Output
- Python Operators
Python Flow Control
- Python if...else Statement
- Python for Loop
- Python while Loop
- Python break and continue
- Python pass Statement
Python Data types
- Python Numbers and Mathematics
- Python List
- Python Tuple
- Python String
- Python Dictionary
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files
- Reading CSV files in Python
- Writing CSV files in Python
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python
- Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
- Precedence and Associativity of Operators in Python
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs
Python Tutorials
Python Dictionary Comprehension
Python Dictionary setdefault()
Python Dictionary clear()
Python Dictionary popitem()
- Python Dictionary update()
- Python Dictionary keys()
Python Nested Dictionary
In Python, a dictionary is an unordered collection of items. For example:
Here, dictionary has a key:value pair enclosed within curly brackets {} .
To learn more about dictionary, please visit Python Dictionary .
- What is Nested Dictionary in Python?
In Python, a nested dictionary is a dictionary inside a dictionary. It's a collection of dictionaries into one single dictionary.
Here, the nested_dict is a nested dictionary with the dictionary dictA and dictB . They are two dictionary each having own key and value.
- Create a Nested Dictionary
We're going to create dictionary of people within a dictionary.
Example 1: How to create a nested dictionary
When we run above program, it will output:
In the above program, people is a nested dictionary. The internal dictionary 1 and 2 is assigned to people . Here, both the dictionary have key name , age , sex with different values. Now, we print the result of people .
- Access elements of a Nested Dictionary
To access element of a nested dictionary, we use indexing [] syntax in Python.
Example 2: Access the elements using the [] syntax
In the above program, we print the value of key name using i.e. people[1]['name'] from internal dictionary 1 . Similarly, we print the value of age and sex one by one.
- Add element to a Nested Dictionary
Example 3: How to change or add elements in a nested dictionary?
In the above program, we create an empty dictionary 3 inside the dictionary people .
Then, we add the key:value pair i.e people[3]['Name'] = 'Luna' inside the dictionary 3 . Similarly, we do this for key age , sex and married one by one. When we print the people[3] , we get key:value pairs of dictionary 3 .
Example 4: Add another dictionary to the nested dictionary
In the above program, we assign a dictionary literal to people[4] . The literal have keys name , age and sex with respective values. Then we print the people[4] , to see that the dictionary 4 is added in nested dictionary people.
- Delete elements from a Nested Dictionary
In Python, we use “ del “ statement to delete elements from nested dictionary.
Example 5: How to delete elements from a nested dictionary?
In the above program, we delete the key:value pairs of married from internal dictionary 3 and 4 . Then, we print the people[3] and people[4] to confirm changes.
Example 6: How to delete dictionary from a nested dictionary?
In the above program, we delete both the internal dictionary 3 and 4 using del from the nested dictionary people . Then, we print the nested dictionary people to confirm changes.
- Iterating Through a Nested Dictionary
Using the for loops, we can iterate through each elements in a nested dictionary.
Example 7: How to iterate through a Nested dictionary?
In the above program, the first loop returns all the keys in the nested dictionary people . It consist of the IDs p_id of each person. We use these IDs to unpack the information p_info of each person.
The second loop goes through the information of each person. Then, it returns all of the keys name , age , sex of each person's dictionary.
Now, we print the key of the person’s information and the value for that key.
Key Points to Remember:
- Nested dictionary is an unordered collection of dictionary
- Slicing Nested Dictionary is not possible.
- We can shrink or grow nested dictionary as need.
- Like Dictionary, it also has key and value.
- Dictionary are accessed using key.
Table of Contents
- Key Points to Remember
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
Python Tutorial
Python Library
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
What is the best way to implement nested dictionaries?
I have a data structure which essentially amounts to a nested dictionary. Let's say it looks like this:
Now, maintaining and creating this is pretty painful; every time I have a new state/county/profession I have to create the lower layer dictionaries via obnoxious try/catch blocks. Moreover, I have to create annoying nested iterators if I want to go over all the values.
I could also use tuples as keys, like such:
This makes iterating over the values very simple and natural, but it is more syntactically painful to do things like aggregations and looking at subsets of the dictionary (e.g. if I just want to go state-by-state).
Basically, sometimes I want to think of a nested dictionary as a flat dictionary, and sometimes I want to think of it indeed as a complex hierarchy. I could wrap this all in a class, but it seems like someone might have done this already. Alternatively, it seems like there might be some really elegant syntactical constructions to do this.
How could I do this better?
Addendum: I'm aware of setdefault() but it doesn't really make for clean syntax. Also, each sub-dictionary you create still needs to have setdefault() manually set.
- data-structures
- autovivification

22 Answers 22
What is the best way to implement nested dictionaries in Python?
This is a bad idea, don't do it. Instead, use a regular dictionary and use dict.setdefault where apropos, so when keys are missing under normal usage you get the expected KeyError . If you insist on getting this behavior, here's how to shoot yourself in the foot:
Implement __missing__ on a dict subclass to set and return a new instance.
This approach has been available (and documented) since Python 2.5, and (particularly valuable to me) it pretty prints just like a normal dict , instead of the ugly printing of an autovivified defaultdict:
(Note self[key] is on the left-hand side of assignment, so there's no recursion here.)
and say you have some data:
Here's our usage code:
A criticism of this type of container is that if the user misspells a key, our code could fail silently:
And additionally now we'd have a misspelled county in our data:
Explanation:
We're just providing another nested instance of our class Vividict whenever a key is accessed but missing. (Returning the value assignment is useful because it avoids us additionally calling the getter on the dict, and unfortunately, we can't return it as it is being set.)
Note, these are the same semantics as the most upvoted answer but in half the lines of code - nosklo's implementation:
class AutoVivification(dict): """Implementation of perl's autovivification feature.""" def __getitem__(self, item): try: return dict.__getitem__(self, item) except KeyError: value = self[item] = type(self)() return value
Demonstration of Usage
Below is just an example of how this dict could be easily used to create a nested dict structure on the fly. This can quickly create a hierarchical tree structure as deeply as you might want to go.
Which outputs:
And as the last line shows, it pretty prints beautifully and in order for manual inspection. But if you want to visually inspect your data, implementing __missing__ to set a new instance of its class to the key and return it is a far better solution.
Other alternatives, for contrast:
Dict.setdefault.
Although the asker thinks this isn't clean, I find it preferable to the Vividict myself.
A misspelling would fail noisily, and not clutter our data with bad information:
Additionally, I think setdefault works great when used in loops and you don't know what you're going to get for keys, but repetitive usage becomes quite burdensome, and I don't think anyone would want to keep up the following:
Another criticism is that setdefault requires a new instance whether it is used or not. However, Python (or at least CPython) is rather smart about handling unused and unreferenced new instances, for example, it reuses the location in memory:
An auto-vivified defaultdict
This is a neat looking implementation, and usage in a script that you're not inspecting the data on would be as useful as implementing __missing__ :
But if you need to inspect your data, the results of an auto-vivified defaultdict populated with data in the same way looks like this:
This output is quite inelegant, and the results are quite unreadable. The solution typically given is to recursively convert back to a dict for manual inspection. This non-trivial solution is left as an exercise for the reader.
Performance
Finally, let's look at performance. I'm subtracting the costs of instantiation.
Based on performance, dict.setdefault works the best. I'd highly recommend it for production code, in cases where you care about execution speed.
If you need this for interactive use (in an IPython notebook, perhaps) then performance doesn't really matter - in which case, I'd go with Vividict for readability of the output. Compared to the AutoVivification object (which uses __getitem__ instead of __missing__ , which was made for this purpose) it is far superior.
Implementing __missing__ on a subclassed dict to set and return a new instance is slightly more difficult than alternatives but has the benefits of
- easy instantiation
- easy data population
- easy data viewing
and because it is less complicated and more performant than modifying __getitem__ , it should be preferred to that method.
Nevertheless, it has drawbacks:
- Bad lookups will fail silently.
- The bad lookup will remain in the dictionary.
Thus I personally prefer setdefault to the other solutions, and have in every situation where I have needed this sort of behavior.
- Excellent answer! Is there any way to specify a finite depth and a leaf type for a Vividict ? E.g. 3 and list for a dict of dict of dict of lists which could be populated with d['primary']['secondary']['tertiary'].append(element) . I could define 3 different classes for each depth but I'd love to find a cleaner solution. – Eric Duminil Commented Dec 27, 2017 at 17:22
- @EricDuminil d['primary']['secondary'].setdefault('tertiary', []).append('element') - ?? Thanks for the compliment, but let me be honest - I never actually use __missing__ - I always use setdefault . I should probably update my conclusion/intro... – Aaron Hall ♦ Commented Dec 27, 2017 at 18:57
- @AaronHall The correct behavior is the code should create a dict if needed. In this case by overriding the previous assigned value. – nehem Commented Mar 13, 2019 at 1:18
- @AaronHall Also can you help me to understand what is meant by The bad lookup will remain in the dictionary. as I'm considering using this solution?. Much appreciated. Thx – nehem Commented Mar 13, 2019 at 2:56
- @AaronHall The problem with it would fail setdefault when it nested more than two levels of depth. Looks like no structure in Python can offer true vivification as described. I had to settle for two stating methods one for get_nested & one for set_nested which accept a reference for dict and list of nested attributes. – nehem Commented Mar 13, 2019 at 4:56
- Anybody have this problem when they moved to python 3.x? stackoverflow.com/questions/54622935/… – jason Commented Feb 11, 2019 at 4:38
- @jason pickle is terrible between python versions. Avoid using it to store data you want to keep. Use it only for caches and stuff you can dump and regenerate at will. Not as a long term storage or serialization method. – nosklo Commented Feb 11, 2019 at 12:09
- What do you use to store these objects? My autovivification object contains just pandas dataframes and string. – jason Commented Feb 11, 2019 at 14:39
- @jason Depending on the data, I like to use JSON, csv files, or even a sqlite database to store it. – nosklo Commented Feb 12, 2019 at 6:37
Just because I haven't seen one this small, here's a dict that gets as nested as you like, no sweat:
- 5 @wberry: Actually all you need is yodict = lambda: defaultdict(yodict) . – martineau Commented Aug 14, 2013 at 18:27
- 1 The accepted version is a subclass of dict , so to be fully equivalent we would need x = Vdict(a=1, b=2) to work. – wberry Commented Aug 15, 2013 at 4:46
- 1 @wberry: Irrespective of what's in the accepted answer, being a subclass of dict wasn't a requirement stated by the OP, who only asked for the "best way" to implement them -- and besides, it doesn't/shouldn't matter that much in Python anyway. – martineau Commented Mar 21, 2014 at 17:43
You could create a YAML file and read it in using PyYaml .
Step 1: Create a YAML file, "employment.yml":
Step 2: Read it in Python
and now my_shnazzy_dictionary has all your values. If you needed to do this on the fly, you can create the YAML as a string and feed that into yaml.safe_load(...) .
- 4 YAML is my definitely my choice for inputting lots of deeply nested data (and configuration files, databaes mockups, etc...). If the OP doesn't want extra files lying around, just use a regular Python string in some file and parse that with YAML. – klozovin Commented Mar 11, 2009 at 20:49
- Good point on creating YAML strings: This would be a much cleaner approach than using the "tempfile" module repeatedly. – Pete Commented Mar 11, 2009 at 20:52
Since you have a star-schema design, you might want to structure it more like a relational table and less like a dictionary.
That kind of thing can go a long way to creating a data warehouse-like design without the SQL overheads.
If the number of nesting levels is small, I use collections.defaultdict for this:
Using defaultdict like this avoids a lot of messy setdefault() , get() , etc.
- +1: defaultdict is one of my all-time favourite additions to python. No more .setdefault()! – John Fouhy Commented Mar 11, 2009 at 23:13
This is a function that returns a nested dictionary of arbitrary depth:
Use it like this:
Iterate through everything with something like this:
This prints out:
You might eventually want to make it so that new items can not be added to the dict. It's easy to recursively convert all these defaultdict s to normal dict s.
As others have suggested, a relational database could be more useful to you. You can use a in-memory sqlite3 database as a data structure to create tables and then query them.
This is just a simple example. You could define separate tables for states, counties and job titles.
I find setdefault quite useful; It checks if a key is present and adds it if not:
setdefault always returns the relevant key, so you are actually updating the values of ' d ' in place.
When it comes to iterating, I'm sure you could write a generator easily enough if one doesn't already exist in Python:
- I like this solution but when I try: count.setdefault(a, {}).setdefault(b, {}).setdefault(c, 0) += 1 I get "illegal expression for augmented assignment" – dfrankow Commented Mar 8, 2011 at 19:31
collections.defaultdict can be sub-classed to make a nested dict. Then add any useful iteration methods to that class.
- 1 This is the answer that comes closest to what I was looking for. But ideally there would be all sorts of helper functions, e.g. walk_keys() or such. I'm surprised there's nothing in the standard libraries to do this. – YGA Commented Mar 14, 2009 at 5:58
You can use Addict: https://github.com/mewwts/addict
As for "obnoxious try/catch blocks":
You can use this to convert from your flat dictionary format to structured format:
defaultdict() is your friend!
For a two dimensional dictionary you can do:
For more dimensions you can:

- This answer works for only three levels at best. For arbitrary levels, consider this answer . – Asclepius Commented Jun 3, 2017 at 21:59
For easy iterating over your nested dictionary, why not just write a simple generator?
So then, if you have your compilicated nested dictionary, iterating over it becomes simple:
Obviously your generator can yield whatever format of data is useful to you.
Why are you using try catch blocks to read the tree? It's easy enough (and probably safer) to query whether a key exists in a dict before trying to retrieve it. A function using guard clauses might look like this:
Or, a perhaps somewhat verbose method, is to use the get method:
But for a somewhat more succinct way, you might want to look at using a collections.defaultdict , which is part of the standard library since python 2.5.
I'm making assumptions about the meaning of your data structure here, but it should be easy to adjust for what you actually want to do.
I like the idea of wrapping this in a class and implementing __getitem__ and __setitem__ such that they implemented a simple query language:
If you wanted to get fancy you could also implement something like:
but mostly I think such a thing would be really fun to implement :D

- I think this is a bad idea -- you can never predict the syntax of keys. You would still override getitem and setitem but have them take tuples. – YGA Commented Mar 11, 2009 at 17:38
- 3 @YGA You're probably right, but it's fun to think about implementing mini languages like this. – Aaron Maenpaa Commented Mar 11, 2009 at 18:25
Unless your dataset is going to stay pretty small, you might want to consider using a relational database. It will do exactly what you want: make it easy to add counts, selecting subsets of counts, and even aggregate counts by state, county, occupation, or any combination of these.
Edit: Now returning dictionaries when querying with wild cards ( None ), and single values otherwise.
- Why return lists? Seems it should either return a dictionary (so you know what each number represents) or a sum (since that's all you can really do with the list). – Ben Blank Commented Mar 11, 2009 at 20:52
I have a similar thing going. I have a lot of cases where I do:
But going many levels deep. It's the ".get(item, {})" that's the key as it'll make another dictionary if there isn't one already. Meanwhile, I've been thinking of ways to deal with this better. Right now, there's a lot of
So instead, I made:
Which has the same effect if you do:
Better? I think so.
You can use recursion in lambdas and defaultdict, no need to define names:
Here's an example:

I used to use this function. its safe, quick, easily maintainable.
For the following (copied from above) is there a way to implement the append function. I am trying to use a nested dictionary to store values as array.
My current implementation is as follows:
I could wrap this all in a class, but it seems like someone might have done this already.
The NestedDict class from the open-source ndicts package (I am the author) tries to mitigate the pain of dealing with nested dictionaries. I think it ticks all the boxes that the questions asks for.
Here you have a summary of its capabilities, for more details check the documentation .
Think of a NestedDict as if it was a flattened dictionary.
At the same time, you can get intermediate nodes, not just the leaf values.
If a key is not present, an exception is thrown.
As in a normal dictionary, if a key is missing it is added to the NestedDict .
This allows to start with an empty NestedDict which can be vivified by setting new items.
When it comes to iteration think of a NestedDict as a flattened dictionary. The familiar .keys() , .values() and .item() methods are available.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python data-structures dictionary mapping autovivification or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Staging Ground Reviewer Motivation
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- Is there a phrase for someone who's really bad at cooking?
- Suitable Category in which Orbit-Stabilizer Theorem Arises Naturally as Canonical Decomposition
- If inflation/cost of living is such a complex difficult problem, then why has the price of drugs been absoultly perfectly stable my whole life?
- How do you end-punctuate quotes when the entire quote is used as a noun phrase?
- I would like to add 9 months onto a field table that is a date and have it populate with the forecast date 9 months later
- Infinite suspension is cotangent complex
- magnetic boots inverted
- What does "seeing from one end of the world to the other" mean?
- Encode a VarInt
- Can you give me an example of an implicit use of Godel's Completeness Theorem, say for example in group theory?
- How can I automatically save my renders with incremental filenames in Blender?
- How did Oswald Mosley escape treason charges?
- Who was the "Dutch author", "Bumstone Bumstone"?
- How can I get the Thevenin equivalent of this circuit?
- Add colored points to QGIS from CSV file of latitude and longitude
- Journal keeps messing with my proof
- Can the SLS's mobile launch platform be rotated at the launch complex to keep the rocket on the leeward side of the tower in case of high winds?
- Is it possible to have a planet that's gaslike in some areas and rocky in others?
- Is 3 Ohm resistance value of a PCB fuse reasonable?
- 3D printed teffilin?
- How to remove obligation to run as administrator in Windows?
- How would you say a couple of letters (as in mail) if they're not necessarily letters?
- 2 in 1: Twin Puzzle
- Where to donate foreign-language academic books?
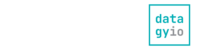
- Learn Python
- Python Lists
- Python Dictionaries
- Python Strings
- Python Functions
- Learn Pandas & NumPy
- Pandas Tutorials
- Numpy Tutorials
- Learn Data Visualization
- Python Seaborn
- Python Matplotlib
Python Nested Dictionary: Complete Guide
- March 25, 2022 December 15, 2022
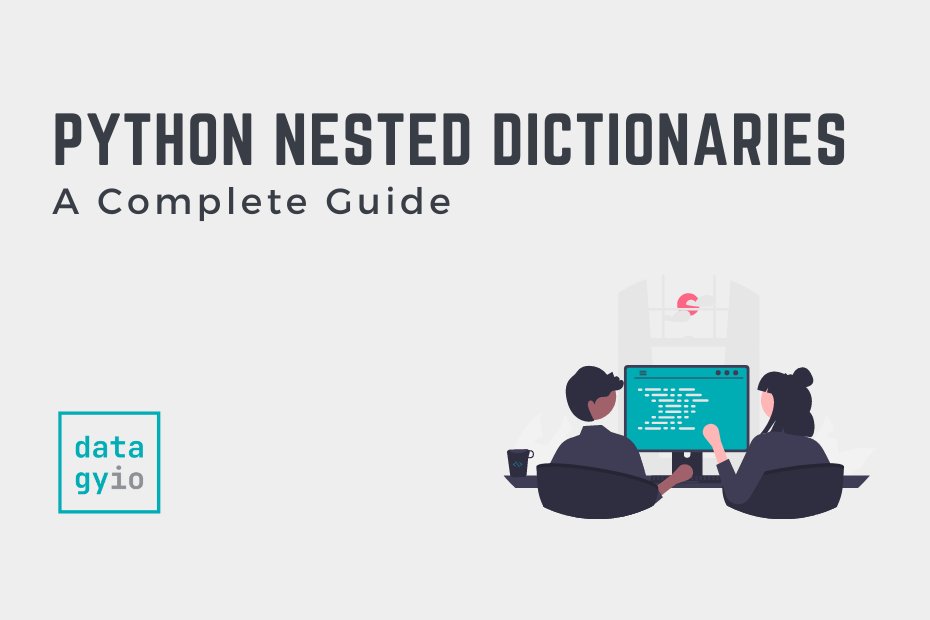
In this tutorial, you’ll learn about Python nested dictionaries – dictionaries that are the values of another dictionary . You’ll learn how to create nested dictionaries, access their elements, modify them and more. You’ll also learn how to work with nested dictionaries to convert them to a Pandas DataFrame.
By the end of this tutorial, you’ll have learned:
- What nested dictionaries are in Python
- How to create nested dictionaries in Python
- How to access, modify, and delete elements in nested dictionaries
- How to convert nested dictionaries to Pandas DataFrames
Table of Contents
Python Nested Dictionaries
Python dictionaries are container data structures that hold key:value pairs of information. They’re created by using curly braces {} , where a value can be looked up by accessing its unique key. Let’s take a look at a simple dictionary example:
In the dictionary above, we have an item called user . We can access its values by referencing the dictionary’s keys. Say we wanted to access the dictionary’s value for the key 'Name' , we could write:
An interesting thing about Python dictionaries is that we can even use other dictionaries as their values. This brings us to the main topic of this article.
Say we wanted to have a dictionary that contained the user information based on someone’s user ID. Let’s create a dictionary that stores the information on multiple users, broken out by an ID:
In this example, our earlier dictionary was embedded into the new, larger dictionary. What we’ve done is created a new, nested dictionary. In the following section, we work through how to create nested dictionaries.
Creating Nested Dictionaries in Python
In this section, you’ll learn how to create nested dictionaries. Nested dictionaries are dictionaries that have another dictionary as one or more of their values . Let’s walk through how we can create Python nested dictionaries.
Let’s take a look at an example:
Let’s break down what we did here:
- We instantiated an empty dictionary, users
- We created two further dictionaries, Nik and Kate
- We then assigned these dictionaries to be the values of the respective keys, 1 and 2 , in the users dictionary
Accessing Items in Nested Dictionaries in Python
In this section, you’ll learn how to access items in a nested Python dictionary. In an earlier section, we explored using the .get() method to access items. Let’s see what happens when we try to access an item in a nested dictionary:
We can see that this returned a dictionary! Since a dictionary was returned we can access an item in the returned dictionary.
Say we wanted to access the 'Name' of user 1 , we could write the following:
We can call the .get() method multiple times since we’re accessing a dictionary within a dictionary. In the next section, you’ll learn how to modify items in a nested dictionary.
Modifying Items in Nested Dictionaries in Python
In this section, you’ll learn how to modify items in nested dictionaries. In order to modify items, we use the same process as we would for setting a new item in a dictionary. In this case, we simply need to navigate a little further down, to a key of a nested dictionary.
Say we wanted to change the profession for user 2 to 'Habitat for Humanity' . We can then write:
In this case, we were able to access the key’s value through direct assignment. If that key didn’t previously exist, then the key (and the value) would have been created.
Deleting Items in Nested Dictionaries in Python
Python dictionaries use the del keyword to delete a key:value pair in a dictionary. In order to do this in a nested dictionary, we simply need to traverse further into the dictionary. Let’s see how we can delete the 'Profession' key from the dictionary 1 :
Similarly, we can delete an entire nested dictionary using the same method. Because the nested dictionary is actually just a key:value pair in our broader dictionary, the same method applies. Let’s delete the entire first dictionary 1 :
In the next section, you’ll learn how to iterate through nested dictionaries in Python.
Iterating Through Nested Dictionaries in Python
In this section, you’ll learn how to iterate through nested dictionaries. This can be helpful when you want to print out the values in the dictionary. We can build a recursive function to handle this. Let’s see what this looks like:
Converting a Nested Python Dictionary to a Pandas DataFrame
In this final section, you’ll learn how to convert a nested dictionary to a Pandas DataFrame. We can simply pass in the nested dictionary into the DataFrame() constructor. However, Pandas will read the DataFrame with the keys as the indices.
To work around this, we can transpose the DataFrame using the .T method :
In this tutorial, you learned about nested dictionaries in Python. You learned what nested dictionaries are. Then you learned how to access, modify, and delete their items. Finally, you learned how to iterate over nested dictionaries as well as how to read them into Pandas DataFrames.
Additional Resources
To learn more about related topics, check out the tutorials below:
- Python Defaultdict: Overview and Examples
- Python: Add Key:Value Pair to Dictionary
- Python: Sort a Dictionary by Values
- Python Merge Dictionaries – Combine Dictionaries (7 Ways)
- Python Dictionaries: Official Documentation
Nik Piepenbreier
Nik is the author of datagy.io and has over a decade of experience working with data analytics, data science, and Python. He specializes in teaching developers how to use Python for data science using hands-on tutorials. View Author posts
8 thoughts on “Python Nested Dictionary: Complete Guide”
Im having a hard time creating a nested dictionary already in a nested group. For starters, created a list and divided the line comments based on a special character. So, I have; [‘nissan’,’altima’,’sedan’] and with many more line items.. Im able to create a dictionary of dict2 = {‘altima’:{‘sedan’,’model below maxima’}. But unable to created the following: Option 1 nest as {‘nissan’:{‘altima’:’sedan’,’model below maxima’} Option 2 dict3 = {‘nissan’,’toyota’} and append the values of dict2 “”nissan related cars” into nissan primary key.
Sorry for my late reply! What are you hoping the dictionary will look like?
I appreciate the insight you have given me here. The challenges am having is that some values in the nested dictionary are list and am to iterate through elements in these list values. Eg d = {‘a1:{‘bk’:[‘aa’,’bbb’,’cc’], ‘pg’:[67,183,213]},’a2′:{‘bk’:[‘zzz’,’mmmm’,’ggg’,’hhh’],’pg’:[300,234,112,,168]}} Am to print out all the elements in the nested dict whose values are list and also count the elements. Expected output: aa bbb cc zzz mmmm ggg hhh 7
Hi Sunday! Thanks for your comment!
You could loop over the dictionary, check the value’s type to see if it’s a list, then print the items and the item’s length. Are you filtering the lists at all?
I’m trying to do exactly what you are and i’m having trouble. Did oyu get a solution?
Hi pv, can you give me more context as to what you’re hoping to do?
Hey there, I’ve got a project with a nested dictionary very similar to yours here. One thing I am trying to do that I can’t quite figure out, is to search for a key, and find others with the same value within the nested dictionary. So to use your example – Give it user #1, who’s profession is ‘datagy,’ I need to find all other users who’s profession is also ‘datagy.’ Does that make sense?
Hey Rod, Thanks for your comment! Do you have an example of what your dictionary looks like? I’d be happy to write some code for you.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python - nested dictionaries, nested dictionaries.
A dictionary can contain dictionaries, this is called nested dictionaries.
Create a dictionary that contain three dictionaries:
Or, if you want to add three dictionaries into a new dictionary:
Create three dictionaries, then create one dictionary that will contain the other three dictionaries:
Access Items in Nested Dictionaries
To access items from a nested dictionary, you use the name of the dictionaries, starting with the outer dictionary:
Print the name of child 2:
Loop Through Nested Dictionaries
You can loop through a dictionary by using the items() method like this:
Loop through the keys and values of all nested dictionaries:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
We Love Servers.
- WHY IOFLOOD?
- BARE METAL CLOUD
- DEDICATED SERVERS
Python Nested Dictionary Guide (With Examples)
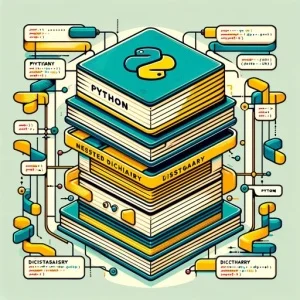
Are you finding it challenging to work with nested dictionaries in Python? You’re not alone. Many developers find themselves tangled in the branches of these complex data structures, but there’s a way to navigate through.
Think of a nested dictionary in Python as a family tree, where each branch can have its own smaller branches. It’s a powerful tool for handling complex data structures, providing a versatile and handy tool for various tasks.
This guide will walk you through the creation, manipulation, and access of nested dictionaries in Python. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering Python nested dictionaries!
TL;DR: How Do I Create and Use a Nested Dictionary in Python?
A nested dictionary in Python is essentially a dictionary within a dictionary. It’s a powerful way to store and organize complex data structures in Python. Here’s a simple example:
In this example, we’ve created a nested dictionary my_dict with two keys: ‘John’ and ‘Jane’. Each key has its own dictionary as a value, containing further key-value pairs. We then access the ‘Age’ of ‘John’ by chaining the keys, resulting in the output ’30’.
This is just a basic way to create and use a nested dictionary in Python. There’s much more to learn about manipulating and accessing data in nested dictionaries. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
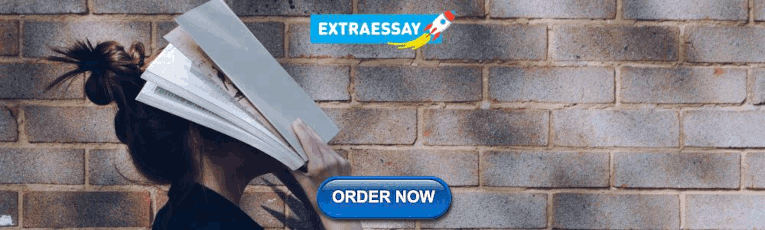
Creating and Manipulating Nested Dictionaries
Advanced techniques with nested dictionaries, exploring alternatives to nested dictionaries, troubleshooting nested dictionaries in python, understanding python’s dictionary data type, python nested dictionaries in real-world applications, wrapping up: mastering python nested dictionaries.
Nested dictionaries in Python allow us to store data in a structured and flexible way. Let’s explore how to create, access, modify, and add new elements to a nested dictionary.
Creating a Nested Dictionary
Creating a nested dictionary is as simple as creating a regular dictionary. The only difference is that the values in a nested dictionary can be dictionaries themselves. Here’s an example:
In this example, we’ve created a nested dictionary my_dict with two keys: ‘John’ and ‘Jane’. Each key has its own dictionary as a value, containing further key-value pairs.
Accessing Elements in a Nested Dictionary
Accessing elements in a nested dictionary involves using the keys from the outer dictionary and the inner dictionary. Here’s how you can do it:
By chaining the keys ‘John’ and ‘Age’, we’re able to access the age of John, which is 30.
Modifying Elements in a Nested Dictionary
Modifying elements in a nested dictionary is done by accessing the specific element using its keys and then assigning a new value to it. Here’s how:
In this example, we’ve changed the age of John from 30 to 31.
Adding New Elements to a Nested Dictionary
Adding new elements to a nested dictionary involves creating a new key in the outer dictionary or in any of the inner dictionaries and assigning a value to it. Here’s an example:
Here, we’ve added a new key-value pair ‘Salary’: 70000 to the dictionary of ‘John’.
Working with nested dictionaries can be tricky, especially for beginners. However, with a bit of practice, you’ll find that they are an incredibly powerful tool for managing complex data structures in Python.
As you become more comfortable with nested dictionaries, you can start to explore more advanced techniques. This includes looping through a nested dictionary, using dictionary comprehension, and applying dictionary methods. Let’s dive into each of these.
Looping Through a Nested Dictionary
Looping through a nested dictionary allows you to access and manipulate each key-value pair in the dictionary. Here’s an example:
In this example, we’re using a nested for loop to iterate over each person’s information in the my_dict nested dictionary. The outer loop iterates over each person, and the inner loop iterates over each piece of information for that person.
Using Dictionary Comprehension with Nested Dictionaries
Dictionary comprehension is a powerful tool that allows you to create new dictionaries in a single, readable line of code. It can also be used with nested dictionaries. Here’s an example:
In this example, we’re using dictionary comprehension to create a new dictionary that contains each person’s age. The expression person: info['Age'] is the key-value pair for the new dictionary, and for person, info in my_dict.items() is the loop.
Using Dictionary Methods with Nested Dictionaries
Python’s dictionary methods can also be used with nested dictionaries. For example, you can use the get() method to safely access the value of a key. If the key doesn’t exist, get() returns None (or a default value of your choice) instead of raising an error:
In this example, we’re trying to access John’s height, which doesn’t exist in the dictionary. Instead of raising a KeyError, the get() method returns ‘Not provided’.
These advanced techniques can help you to work more effectively with nested dictionaries in Python, allowing you to write more efficient and readable code.
While nested dictionaries are a powerful tool for handling complex data structures in Python, they are not the only solution. Alternatives such as classes or namedtuples can also be used, each with their own advantages and drawbacks. Let’s explore these alternatives.
Using Classes Instead of Nested Dictionaries
Classes in Python provide a way of bundling data and functionality together. They can be a more organized and readable alternative to nested dictionaries, especially when dealing with complex data structures:
In this example, we’ve created a Person class with attributes for name , age , and job . We then create two instances of the class, John and Jane . We can access the age of John simply by using John.age .
The advantage of using classes is that they provide a clear structure to the data and can bundle related functionality. However, they can be overkill for simple data structures and require more code to set up.
Using Namedtuples Instead of Nested Dictionaries
Namedtuples are a subclass of Python’s built-in tuple data type. They are immutable and can be a more memory-efficient alternative to nested dictionaries:
In this example, we’ve created a Person namedtuple with fields for name , age , and job . We then create two instances of the namedtuple, John and Jane . We can access the age of John simply by using John.age .
The advantage of using namedtuples is that they are more memory-efficient and can be used as dictionary keys. However, they are immutable, meaning that once a namedtuple is created, it cannot be modified.
When deciding between nested dictionaries, classes, or namedtuples, consider the complexity of your data structure, the memory efficiency, and whether you need the data to be mutable or immutable.
Working with nested dictionaries in Python is not without its challenges. Let’s discuss some common issues you may encounter, such as KeyError, and how to resolve them.
Dealing with KeyError
One of the most common issues when working with nested dictionaries is a KeyError. This error occurs when you try to access a key that doesn’t exist in the dictionary. Here’s an example:
In this example, we’re trying to access the ‘Height’ of ‘John’, which doesn’t exist in the dictionary. This would normally raise a KeyError, but we’ve used a try-except block to catch the error and print a friendly message instead.
Tips for Working with Nested Dictionaries
Here are some tips to help you work more effectively with nested dictionaries in Python:
- Use the get() method : As we’ve seen earlier, the get() method is a safe way to access a key’s value without raising an error if the key doesn’t exist.
- Check if a key exists : You can check if a key exists in a dictionary before trying to access its value using the in keyword.
- Use a default dictionary : Python’s collections module has a defaultdict class that you can use to provide a default value for keys that don’t exist in the dictionary.
Working with nested dictionaries can be tricky, but with these tips and a bit of practice, you’ll be able to handle them effectively.
Before we dive deeper into nested dictionaries, it’s essential to understand the basics of Python’s dictionary data type and why it’s such a powerful tool for handling data.
Python’s Dictionary Data Type
A dictionary in Python is an unordered collection of items. Each item in a dictionary is stored as a key-value pair. Here’s a simple example:
In this example, we’ve created a dictionary simple_dict with three key-value pairs. We can access the value of a key by using the key inside square brackets [] .
Why Dictionaries Are Useful
Dictionaries are incredibly versatile and efficient for data storage and retrieval. They allow you to store data in a way that’s easy to access and modify. Unlike lists or tuples, which use indices, dictionaries use keys, making it easier to retrieve values without needing to know their position.
The Concept of Nesting in Python
Nesting is a concept in Python where a data structure is used as an element of another data structure. For instance, a list can have other lists as its elements, and a dictionary can have other dictionaries as its values. This concept allows you to create complex data structures.
Nested Dictionaries: A Powerful Tool
Nested dictionaries take the power of dictionaries to a whole new level. They allow you to create complex data structures within a single dictionary, making it easier to organize and access data. As we’ve seen in the previous sections, you can create, access, modify, and loop through a nested dictionary in much the same way as a regular dictionary.
Understanding the fundamentals of Python’s dictionary data type and the concept of nesting will help you better understand and utilize nested dictionaries.
Nested dictionaries in Python are not just a theoretical concept — they have practical applications in various fields such as data analysis and web development.
Nested Dictionaries in Data Analysis
In data analysis, nested dictionaries can be used to store and manipulate complex data structures. For example, you might have a dataset where each entry consists of several attributes, and each attribute can have its own set of sub-attributes. A nested dictionary can conveniently store this data in a structured manner.
Nested Dictionaries in Web Development
In web development, nested dictionaries are often used to handle JSON data. JSON (JavaScript Object Notation) is a popular data format for exchanging data between a server and a web application. Since JSON data is structured as key-value pairs, it maps directly to a dictionary in Python. If the JSON data is complex, it can be represented as a nested dictionary.
Handling JSON Data in Python
Python has built-in support for JSON data. You can convert a JSON string into a dictionary using the json.loads() function, and convert a dictionary into a JSON string using the json.dumps() function. Here’s an example:
In this example, we first convert a JSON string into a nested dictionary using json.loads() . We then access the age of John, which is 30. Finally, we convert the dictionary back into a JSON string using json.dumps() .
Further Resources for Mastering Python Nested Dictionaries
If you want to dive deeper into nested dictionaries in Python, here are some resources that you might find useful:
- Mastering Python Dictionary Operations with IOFlood – Master Python dictionary syntax and become proficient in using this essential data structure.
Keeping Your Dictionaries Current: Python Update Dictionary – Master the art of updating dictionaries in Python.
Sorting Python Dictionaries by Value: A Step-by-Step Tutorial – Learn how to sort a Python dictionary by its values.
Python Documentation: Data Structures – The official Python documentation provides a thorough overview of dictionaries, including nested dictionaries.
Real Python: Dictionaries in Python – This article from Real Python goes in-depth on dictionaries and includes several examples and exercises.
Python Course: Advanced Dictionaries – This tutorial covers advanced topics on dictionaries, including dictionary comprehensions and nested dictionaries.
In this comprehensive guide, we’ve delved deep into the world of Python nested dictionaries, a powerful tool for managing complex data structures.
We began with the basics, learning how to create, access, modify, and add new elements to a nested dictionary. We then ventured into more advanced territory, exploring techniques such as looping through a nested dictionary, using dictionary comprehension, and applying dictionary methods.
Along the way, we tackled common challenges you might face when working with nested dictionaries, such as KeyError, providing you with solutions and tips for each issue.
We also looked at alternative approaches to handling complex data structures, comparing nested dictionaries with other data structures like classes and namedtuples.
Here’s a quick comparison of these methods:
Method | Flexibility | Memory Efficiency | Ease of Use |
---|---|---|---|
Nested Dictionaries | High | Moderate | High |
Classes | High | Low | Moderate |
Namedtuples | Low | High | High |
Whether you’re just starting out with Python nested dictionaries or you’re looking to level up your data management skills, we hope this guide has given you a deeper understanding of nested dictionaries and their capabilities.
With their balance of flexibility, memory efficiency, and ease of use, nested dictionaries are a powerful tool for managing complex data structures in Python. Now, you’re well equipped to navigate the branches of Python nested dictionaries. Happy coding!
About Author

Gabriel Ramuglia
Gabriel is the owner and founder of IOFLOOD.com , an unmanaged dedicated server hosting company operating since 2010.Gabriel loves all things servers, bandwidth, and computer programming and enjoys sharing his experience on these topics with readers of the IOFLOOD blog.
Related Posts
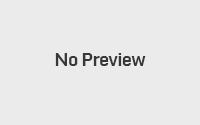
Python Nested Dictionary
A nested dictionary is a dictionary where each key can map to another dictionary rather than a simple value like an integer or string. This can be extended to multiple levels, creating a hierarchy of dictionaries. A basic nested dictionary looks like this:
Nested dictionaries excel at representing data with multiple layers of organization. Some common use cases include:
- Employee Records : Organize employees by department, with each employee’s details in a nested dictionary.
- Inventory Systems : Track product categories, subcategories, and individual product details.
- JSON Data : Nested dictionaries naturally map to the structure of JSON data, making them essential for working with APIs and web services.
In this tutorial, we’ll explore how to create, access, and manipulate nested dictionaries in Python.
Creating a Nested Dictionary
Direct creation.
The most straightforward way to create a nested dictionary is to specify dictionaries as the values for the keys within curly braces.
Let’s create a nested dictionary to store employee records:
Using dict() Constructor
You can also create a nested dictionary using the dict() constructor. Simply provide the key-value pairs as keyword arguments to dict() function.
This method is useful if you have the key-value pairs ready. However, if you have separate lists for keys (e.g., employee IDs) and values (e.g., dictionaries of employee info), you can combine them using zip() and pass them to dict() .
Here, zip() combines the IDs and EmpInfo lists, creating pairs that dict() then converts into a nested dictionary.
Creating a Nested Dictionary with Default Values
Sometimes, you might need a nested dictionary with default values. In such cases, use the fromkeys() method. This method takes an iterable of keys and a default value, which is a dictionary in our case. The result is a nested dictionary with the given keys and the specified default dictionary as the value for each key.
This generates a nested dictionary D where the keys are employee IDs, and each value is a dictionary containing empty strings for ‘name’ and ‘job’.
Accessing Elements in a Nested Dictionary
You can access elements within a nested dictionary by specifying multiple keys in a chain, using square brackets [] . Each key represents a level of nesting.
Attempting to access a key that does not exist within the nested dictionary will raise a KeyError .
To prevent this exception, you can use the get() method. This method returns the value for key if key is in the dictionary, else None, so that this method never raises a KeyError .
Modifying Elements in a Nested Dictionary
Modifying elements within a nested dictionary is straightforward. Just as you use keys to access specific values, you can also use them to change those values.
Adding Elements to a Nested Dictionary
You can easily add new keys at any level of nesting by directly assigning a value to the new key.
Merging Nested Dictionaries
Merging nested dictionaries can be done in two ways, shallow merging and deep merging, depending on the desired outcome.
Shallow Merge
Shallow merging is a straightforward approach where the update() method is used. This method simply adds key-value pairs from one dictionary to another. For example:
However, it is important to note that this is a shallow merge, meaning that if there are overlapping keys, the values from the second dictionary will overwrite the values in the first.
Notice how the ‘name’ and ‘job’ keys from D1 are lost in the merged dictionary because they were overwritten by the ‘salary’ key from D2 .
To overcome this, we can employ deep merging. This method recursively merges dictionaries at all levels, ensuring that values are combined rather than overwritten. A common way to implement deep merging is to use a recursive function like this:
In this example, the deep_update function iterates through the keys and values of the second dictionary. If a value is itself a dictionary (a collections.abc.Mapping ), the function recursively calls itself to merge the nested dictionaries. Otherwise, it simply updates the value in the source dictionary. This results in a complete merging of the dictionaries at all levels.
Removing Nested Dictionary Elements
There are several ways to remove elements from a nested dictionary.
Remove an Item by Key
If you know the key of the item you want to remove, the pop() method is a convenient tool. It not only removes the key-value pair but also returns the removed value.
If you don’t need the removed value, the del statement can be used. It simply removes the key-value pair based on the specified key.
Remove Last Inserted Item
In cases where you want to remove the last inserted item, the popitem() method comes in handy. It removes and returns the last inserted item as a tuple containing the key and value.
It’s important to note that in Python versions before 3.7, the behavior of popitem() was to remove a random item, not necessarily the last inserted one.
Iterating Through a Nested Dictionary
You can iterate through a nested dictionary using nested loops. Here’s an example of iterating through both the outer and inner dictionaries:
In this example, the outer loop iterates over each item in the main dictionary D , while the inner loop iterates over each key-value pair within the nested dictionary info .
Checking for Existence of Keys
To verify if a specific key exists within a nested dictionary, you can utilize the in operator. This operator allows you to check for the presence of a key at any level of the nested structure.
Working with Nested Dictionaries in Python: A Guide
Introduction to nested dictionaries in python.
Nested dictionaries in Python are dictionaries containing other dictionaries as values. This data structure is useful for organizing and managing complex data, such as configuration settings or JSON data. In this article, we will walk through the properties of nested dictionaries, their usage, and real-life examples of how they can be efficiently utilized in Python code.
Properties and Usage of Nested Dictionaries
A nested dictionary, like any other dictionary, can have keys and values of different data types (strings, numbers, lists, etc.). However, the unique property of nested dictionaries is that their values are also dictionaries, which can have their own keys and values. Nested dictionaries are ideal for representing hierarchical or relational data.
The key properties and usage patterns of nested dictionaries include:
Accessing nested dictionary data : Use a series of keys in brackets to access data within the nested dictionaries, e.g., nested_dict['key1']['key2'] .
Adding and updating values : You can add new key-value pairs and update existing ones in the nested dictionaries using the assignment operator = .
Deleting keys : Remove keys and their associated values from a nested dictionary using Python’s del statement.
Looping through nested dictionaries : Use nested for loops and the items() method to iterate through the nested dictionary and access keys and values at different levels of depth.
Simplified Real-life Example
Let’s assume we have an application that manages user profiles. Each user profile consists of a username, contact information, and preferences. We can represent this data structure in Python using nested dictionaries, as shown in this example:
Complex Real-life Example
Imagine an application that stores information about scientific publications: their authors, publication year, and a list of quotes. We can represent this data in Python using a nested dictionary. While there are other ways to achieve this (e.g., using classes), this example illustrates the versatility of nested dictionaries.
Personal Tips on Nested Dictionaries
Use helper functions : When working with complex nested dictionaries, helper functions can simplify code, make it more readable, and reduce errors.
Efficient key search : When searching for a specific key, the get() method can be useful in nested dictionaries to avoid errors and provide default values.
Consider alternative data structures : Although nested dictionaries are convenient for representing complex data, it’s crucial to consider other data structures like classes or namedtuple, which can provide better code organization, reuse, and maintainability.
JSON compatibility : Nested dictionaries can easily be converted to JSON format using the json module. This can be beneficial for interoperability with other systems and languages.
Avoid overly complex nests : While nested dictionaries can be powerful, overly complex nests might signal that alternative data structures should be considered to maintain code readability and manageability.
Stay up to date with our latest content - No spam!
Related Posts
Appending data to csv files with python: a guide for developers.
Learn how to efficiently append data to a CSV file using Python, with examples and best practices for handling large datasets and complex structures.
Calculating the Sum of Elements in a Python List
Learn how to calculate the sum of elements in a Python list easily and efficiently using built-in methods and your own custom functions.
Comparing Multiple Lists in Python: Methods & Techniques
Compare multiple lists in Python efficiently with various techniques, including set operations, list comprehensions, and built-in functions.
Comparing Multiple Objects in Python: A Guide for Developers
Compare multiple objects in Python using built-in functions and custom solutions for efficient code. Boost your Python skills with this easy guide.
- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Define a Nested Dictionary in Python
In Python, dictionaries are powerful data structures that allow you to store and organize data using key-value pairs. When you need to represent complex and hierarchical data, nested dictionaries come in handy. A nested dictionary is a dictionary that contains one or more dictionaries as values. In this article, we will explore some simple methods to define a nested dictionary in Python.
How to Define a Nested Dictionary in Python?
Below, are the methods for How to Define a Nested Dictionary in Python .
- Using Curly Braces
- Using a For Loop
- Using dict() Constructor
- Using defaultdict
Define a Nested Dictionary Using Curly Braces
The most straightforward way to create a nested dictionary is by using curly braces {} . You can nest dictionaries by placing one set of curly braces inside another. In this example, the outer dictionary has a key ‘outer_key’ with a corresponding value that is another dictionary with keys ‘inner_key1’ and ‘inner_key2’.
Define a Nested Dictionary in Python Using a For Loop
If you have a large number of nested keys or want to dynamically create a nested dictionary, you can use loops to build the structure. This method allows you to iterate through lists of outer and inner keys, dynamically creating a nested dictionary structure.
Define a Nested Dictionary Using dict() Constructor
Another method to create a nested dictionary is by using the dict() constructor and specifying key-value pairs for the inner dictionaries. This method provides a more explicit way to define nested dictionaries by calling the dict() constructor for the outer dictionary and providing key-value pairs for the inner dictionaries.
Define a Nested Dictionary Using defaultdict
The defaultdict class from the collections module can be employed to create a nested dictionary with default values for missing keys. This is particularly useful when you plan to update the inner dictionaries later. In this example, the defaultdict is initialized with the default value type of dict . It automatically creates inner dictionaries for each outer key, allowing you to append values to them.
Please Login to comment...
Similar reads.
- Python Programs
- Python-nested-dictionary
- Best 10 IPTV Service Providers in Germany
- Python 3.13 Releases | Enhanced REPL for Developers
- IPTV Anbieter in Deutschland - Top IPTV Anbieter Abonnements
- Best SSL Certificate Providers in 2024 (Free & Paid)
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
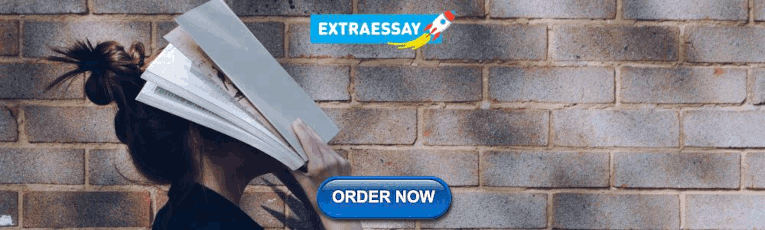
IMAGES
VIDEO
COMMENTS
I need to assign nested values to a dictionary. I have simplified my question for ease of understanding: Data = {} day1 = 'March12'. day2 = 'March14'. e1 = 'experiment1'. e2 = 'experiment2'. Data[day1][e1] = 4. But the Data[day1][e1] = 4 command does not function (for the same reason as test = {} ; test["foo"]["bar"] = 0 ).
In this article, you’ll learn about nested dictionary in Python. More specifically, you’ll learn to create nested dictionary, access elements, modify them and so on with the help of examples.
What is the best way to implement nested dictionaries in Python? This is a bad idea, don't do it. Instead, use a regular dictionary and use dict.setdefault where apropos, so when keys are missing under normal usage you get the expected KeyError. If you insist on getting this behavior, here's how to shoot yourself in the foot:
In Python, a Nested dictionary can be created by placing the comma-separated dictionaries enclosed within braces. Python3. Dict = { 'Dict1': { }, 'Dict2': { }} print("Nested dictionary 1-") print(Dict) Dict = { 'Dict1': {'name': 'Ali', 'age': '19'}, 'Dict2': {'name': 'Bob', 'age': '25'}}
In this tutorial, you’ll learn about Python nested dictionaries – dictionaries that are the values of another dictionary. You’ll learn how to create nested dictionaries, access their elements, modify them and more. You’ll also learn how to work with nested dictionaries to convert them to a Pandas DataFrame.
Nested Dictionaries. A dictionary can contain dictionaries, this is called nested dictionaries. Example Get your own Python Server. Create a dictionary that contain three dictionaries: myfamily = { "child1" : { "name" : "Emil", "year" : 2004. }, "child2" : { "name" : "Tobias", "year" : 2007. }, "child3" : { "name" : "Linus", "year" : 2011. }
This guide will walk you through the creation, manipulation, and access of nested dictionaries in Python. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches. So, let’s dive in and start mastering Python nested dictionaries! TL;DR: How Do I Create and Use a Nested Dictionary in Python?
Learn to create a Nested Dictionary in Python, access change add and remove nested dictionary items, iterate through a nested dictionary and more.
Adding and updating values: You can add new key-value pairs and update existing ones in the nested dictionaries using the assignment operator =. Deleting keys: Remove keys and their associated values from a nested dictionary using Python’s del statement.
This method provides a more explicit way to define nested dictionaries by calling the dict() constructor for the outer dictionary and providing key-value pairs for the inner dictionaries. Python3. nested_dict = dict(outer_key=dict(inner_key1='value1', inner_key2='value2'))