
- PHP All Exercises & Assignments
Practice your PHP skills using PHP Exercises & Assignments. Tutorials Class provides you exercises on PHP basics, variables, operators, loops, forms, and database.
Once you learn PHP, it is important to practice to understand PHP concepts. This will also help you with preparing for PHP Interview Questions.
Here, you will find a list of PHP programs, along with problem description and solution. These programs can also be used as assignments for PHP students.
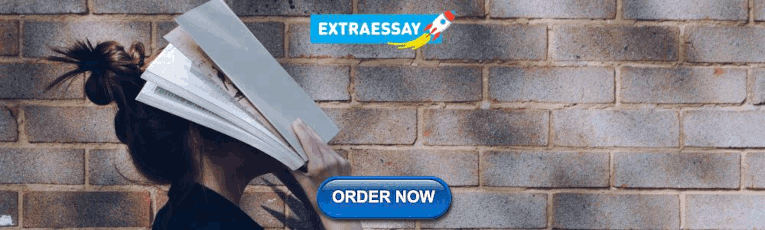
Write a program to count 5 to 15 using PHP loop
Description: Write a Program to display count, from 5 to 15 using PHP loop as given below.
Rules & Hint
- You can use “for” or “while” loop
- You can use variable to initialize count
- You can use html tag for line break
View Solution/Program
5 6 7 8 9 10 11 12 13 14 15
Write a program to print “Hello World” using echo
Description: Write a program to print “Hello World” using echo only?
Conditions:
- You can not use any variable.
View Solution /Program
Hello World
Write a program to print “Hello PHP” using variable
Description: Write a program to print “Hello PHP” using php variable?
- You can not use text directly in echo but can use variable.
Write a program to print a string using echo+variable.
Description: Write a program to print “Welcome to the PHP World” using some part of the text in variable & some part directly in echo.
- You have to use a variable that contains string “PHP World”.
Welcome to the PHP World
Write a program to print two variables in single echo
Description: Write a program to print 2 php variables using single echo statement.
- First variable have text “Good Morning.”
- Second variable have text “Have a nice day!”
- Your output should be “Good morning. Have a nice day!”
- You are allowed to use only one echo statement in this program.
Good Morning. Have a nice day!
Write a program to check student grade based on marks
Description:.
Write a program to check student grade based on the marks using if-else statement.
- If marks are 60% or more, grade will be First Division.
- If marks between 45% to 59%, grade will be Second Division.
- If marks between 33% to 44%, grade will be Third Division.
- If marks are less than 33%, student will be Fail.
Click to View Solution/Program
Third Division
Write a program to show day of the week using switch
Write a program to show day of the week (for example: Monday) based on numbers using switch/case statements.
- You can pass 1 to 7 number in switch
- Day 1 will be considered as Monday
- If number is not between 1 to 7, show invalid number in default
It is Friday!
Write a factorial program using for loop in php
Write a program to calculate factorial of a number using for loop in php.
The factorial of 3 is 6
Factorial program in PHP using recursive function
Exercise Description: Write a PHP program to find factorial of a number using recursive function .
What is Recursive Function?
- A recursive function is a function that calls itself.
Write a program to create Chess board in PHP using for loop
Write a PHP program using nested for loop that creates a chess board.
- You can use html table having width=”400px” and take “30px” as cell height and width for check boxes.

Write a Program to create given pattern with * using for loop
Description: Write a Program to create following pattern using for loops:
- You can use for or while loop
- You can use multiple (nested) loop to draw above pattern
View Solution/Program using two for loops
* ** *** **** ***** ****** ******* ********
Simple Tips for PHP Beginners
When a beginner start PHP programming, he often gets some syntax errors. Sometimes these are small errors but takes a lot of time to fix. This happens when we are not familiar with the basic syntax and do small mistakes in programs. These mistakes can be avoided if you practice more and taking care of small things.
I would like to say that it is never a good idea to become smart and start copying. This will save your time but you would not be able to understand PHP syntax. Rather, Type your program and get friendly with PHP code.
Follow Simple Tips for PHP Beginners to avoid errors in Programming
- Start with simple & small programs.
- Type your PHP program code manually. Do not just Copy Paste.
- Always create a new file for new code and keep backup of old files. This will make it easy to find old programs when needed.
- Keep your PHP files in organized folders rather than keeping all files in same folder.
- Use meaningful names for PHP files or folders. Some examples are: “ variable-test.php “, “ loops.php ” etc. Do not just use “ abc.php “, “ 123.php ” or “ sample.php “
- Avoid space between file or folder names. Use hyphens (-) instead.
- Use lower case letters for file or folder names. This will help you make a consistent code
These points are not mandatory but they help you to make consistent and understandable code. Once you practice this for 20 to 30 PHP programs, you can go further with more standards.
The PHP Standard Recommendation (PSR) is a PHP specification published by the PHP Framework Interop Group.
Experiment with Basic PHP Syntax Errors
When you start PHP Programming, you may face some programming errors. These errors stops your program execution. Sometimes you quickly find your solutions while sometimes it may take long time even if there is small mistake. It is important to get familiar with Basic PHP Syntax Errors
Basic Syntax errors occurs when we do not write PHP Code correctly. We cannot avoid all those errors but we can learn from them.
Here is a working PHP Code example to output a simple line.
Output: Hello World!
It is better to experiment with PHP Basic code and see what errors happens.
- Remove semicolon from the end of second line and see what error occurs
- Remove double quote from “Hello World!” what error occurs
- Remove PHP ending statement “?>” error occurs
- Use “
- Try some space between “
Try above changes one at a time and see error. Observe What you did and what error happens.
Take care of the line number mentioned in error message. It will give you hint about the place where there is some mistake in the code.
Read Carefully Error message. Once you will understand the meaning of these basic error messages, you will be able to fix them later on easily.
Note: Most of the time error can be found in previous line instead of actual mentioned line. For example: If your program miss semicolon in line number 6, it will show error in line number 7.
Using phpinfo() – Display PHP Configuration & Modules
phpinfo() is a PHP built-in function used to display information about PHP’s configuration settings and modules.
When we install PHP, there are many additional modules also get installed. Most of them are enabled and some are disabled. These modules or extensions enhance PHP functionality. For example, the date-time extension provides some ready-made function related to date and time formatting. MySQL modules are integrated to deal with PHP Connections.
It is good to take a look on those extensions. Simply use
phpinfo() function as given below.
Example Using phpinfo() function

Write a PHP program to add two numbers
Write a program to perform sum or addition of two numbers in PHP programming. You can use PHP Variables and Operators
PHP Program to add two numbers:
Write a program to calculate electricity bill in php.
You need to write a PHP program to calculate electricity bill using if-else conditions.
- For first 50 units – Rs. 3.50/unit
- For next 100 units – Rs. 4.00/unit
- For next 100 units – Rs. 5.20/unit
- For units above 250 – Rs. 6.50/unit
- You can use conditional statements .
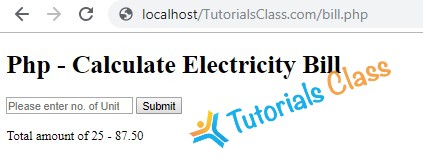
Write a simple calculator program in PHP using switch case
You need to write a simple calculator program in PHP using switch case.
Operations:
- Subtraction
- Multiplication

Remove specific element by value from an array in PHP?
You need to write a program in PHP to remove specific element by value from an array using PHP program.
Instructions:
- Take an array with list of month names.
- Take a variable with the name of value to be deleted.
- You can use PHP array functions or foreach loop.
Solution 1: Using array_search()
With the help of array_search() function, we can remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [3]=> string(5) “april” [4]=> string(3) “may” }
Solution 2: Using foreach()
By using foreach() loop, we can also remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [3]=> string(5) “april” [4]=> string(3) “may” }
Solution 3: Using array_diff()
With the help of array_diff() function, we also can remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [2]=> string(5) “march” [4]=> string(3) “may” }
Write a PHP program to check if a person is eligible to vote
Write a PHP program to check if a person is eligible to vote or not.
- Minimum age required for vote is 18.
- You can use PHP Functions .
- You can use Decision Making Statements .
Click to View Solution/Program.
You Are Eligible For Vote
Write a PHP program to calculate area of rectangle
Write a PHP program to calculate area of rectangle by using PHP Function.
- You must use a PHP Function .
- There should be two arguments i.e. length & width.
View Solution/Program.
Area Of Rectangle with length 2 & width 4 is 8 .
- Next »
- PHP Exercises Categories
- PHP Top Exercises
- PHP Variables
- PHP Decision Making
- PHP Functions
- PHP Operators
- Language Reference
Assignment Operators
The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left operand gets set to the value of the expression on the right (that is, "gets set to").
The value of an assignment expression is the value assigned. That is, the value of " $a = 3 " is 3. This allows you to do some tricky things: <?php $a = ( $b = 4 ) + 5 ; // $a is equal to 9 now, and $b has been set to 4. ?>
In addition to the basic assignment operator, there are "combined operators" for all of the binary arithmetic , array union and string operators that allow you to use a value in an expression and then set its value to the result of that expression. For example: <?php $a = 3 ; $a += 5 ; // sets $a to 8, as if we had said: $a = $a + 5; $b = "Hello " ; $b .= "There!" ; // sets $b to "Hello There!", just like $b = $b . "There!"; ?>
Note that the assignment copies the original variable to the new one (assignment by value), so changes to one will not affect the other. This may also have relevance if you need to copy something like a large array inside a tight loop.
An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference. Objects may be explicitly copied via the clone keyword.
Assignment by Reference
Assignment by reference is also supported, using the " $var = &$othervar; " syntax. Assignment by reference means that both variables end up pointing at the same data, and nothing is copied anywhere.
Example #1 Assigning by reference
The new operator returns a reference automatically, as such assigning the result of new by reference is an error.
The above example will output:
More information on references and their potential uses can be found in the References Explained section of the manual.
Arithmetic Assignment Operators
Example | Equivalent | Operation |
---|---|---|
$a += $b | $a = $a + $b | Addition |
$a -= $b | $a = $a - $b | Subtraction |
$a *= $b | $a = $a * $b | Multiplication |
$a /= $b | $a = $a / $b | Division |
$a %= $b | $a = $a % $b | Modulus |
$a **= $b | $a = $a ** $b | Exponentiation |
Bitwise Assignment Operators
Example | Equivalent | Operation |
---|---|---|
$a &= $b | $a = $a & $b | Bitwise And |
$a |= $b | $a = $a | $b | Bitwise Or |
$a ^= $b | $a = $a ^ $b | Bitwise Xor |
$a <<= $b | $a = $a << $b | Left Shift |
$a >>= $b | $a = $a >> $b | Right Shift |
Other Assignment Operators
Example | Equivalent | Operation |
---|---|---|
$a .= $b | $a = $a . $b | String Concatenation |
$a ??= $b | $a = $a ?? $b | Null Coalesce |
- arithmetic operators
- bitwise operators
- null coalescing operator
Improve This Page
User contributed notes.

PHP Assignment Operators
Php tutorial index.
PHP assignment operators applied to assign the result of an expression to a variable. = is a fundamental assignment operator in PHP. It means that the left operand gets set to the value of the assignment expression on the right.
Operator | Description |
---|---|
= | Assign |
+= | Increments then assigns |
-= | Decrements then assigns |
*= | Multiplies then assigns |
/= | Divides then assigns |
%= | Modulus then assigns |
- TutorialKart
- SAP Tutorials
- Salesforce Admin
- Salesforce Developer
- Visualforce
- Informatica
- Kafka Tutorial
- Spark Tutorial
- Tomcat Tutorial
- Python Tkinter
Programming
- Bash Script
- Julia Tutorial
- CouchDB Tutorial
- MongoDB Tutorial
- PostgreSQL Tutorial
- Android Compose
- Flutter Tutorial
- Kotlin Android
Web & Server
- Selenium Java
- PHP Tutorial
- PHP Install
- PHP Hello World
- Check if variable is set
- Convert string to int
- Convert string to float
- Convert string to boolean
- Convert int to string
- Convert int to float
- Get type of variable
- Subtraction
- Multiplication
- Exponentiation
- Simple Assignment
- Addition Assignment
- Subtraction Assignment
- ADVERTISEMENT
- Multiplication Assignment
- Division Assignment
- Modulus Assignment
- PHP Comments
- Python If AND
- Python If OR
- Python If NOT
- Do-While Loop
- Nested foreach
- echo() vs print()
- printf() vs sprintf()
- PHP Strings
- Create a string
- Create empty string
- Compare strings
- Count number of words in string
- Get ASCII value of a character
- Iterate over characters of a string
- Iterate over words of a string
- Print string to console
- String length
- Substring of a string
- Check if string is empty
- Check if strings are equal
- Check if strings are equal ignoring case
- Check if string contains specified substring
- Check if string starts with specific substring
- Check if string ends with specific substring
- Check if string starts with specific character
- Check if string starts with http
- Check if string starts with a number
- Check if string starts with an uppercase
- Check if string starts with a lowercase
- Check if string ends with a punctuation
- Check if string ends with forward slash (/) character
- Check if string contains number(s)
- Check if string contains only alphabets
- Check if string contains only numeric digits
- Check if string contains only lowercase
- Check if string contains only uppercase
- Check if string value is a valid number
- Check if string is a float value
- Append a string with suffix string
- Prepend a string with prefix string
- Concatenate strings
- Concatenate string and number
- Insert character at specific index in string
- Insert substring at specific index in string
- Repeat string for N times
- Replace substring
- Replace first occurrence
- Replace last occurrence
- Trim spaces from edges of string
- Trim specific characters from edges of the string
- Split string
- Split string into words
- Split string by comma
- Split string by single space
- Split string by new line
- Split string by any whitespace character
- Split string by one or more whitespace characters
- Split string into substrings of specified length
- Transformations
- Reverse a string
- Convert string to lowercase
- Convert string to uppercase
- Join elements of string array with separator string
- Delete first character of string
- Delete last character of string
- Count number of occurrences of substring in a string
- Count alphabet characters in a string
- Find index of substring in a string
- Find index of last occurrence in a string
- Find all the substrings that match a pattern
- Check if entire string matches a regular expression
- Check if string has a match for regular expression
- Sort array of strings
- Sort strings in array based on length
- Foramatting
- PHP – Format string
- PHP – Variable inside a string
- PHP – Parse JSON String
- Conversions
- PHP – Convert CSV String into Array
- PHP – Convert string array to CSV string
- Introduction to Arrays
- Indexed arrays
- Associative arrays
- Multi-dimensional arrays
- String array
- Array length
- Create Arrays
- Create indexed array
- Create associative array
- Create empty array
- Check if array is empty
- Check if specific element is present in array .
- Check if two arrays are equal
- Check if any two adjacent values are same
- Read/Access Operations
- Access array elements using index
- Iterate through array using For loop
- Iterate over key-value pairs using foreach
- Array foreach()
- Get first element in array
- Get last element in array
- Get keys of array
- Get index of a key in array
- Find Operations
- Count occurrences of specific value in array
- Find index of value in array
- Find index of last occurrence of value in array
- Combine two arrays to create an Associative Array
- Convert array to string
- Convert array to CSV string
- Join array elements
- Reverse an array
- Split array into chunks
- Slice array by index
- Slice array by key
- Preserve keys while slicing array
- Truncate array
- Remove duplicate values in array
- Filter elements in array based on a condition
- Filter positive numbers in array
- Filter negative numbers in array
- Remove empty strings in array
- Delete Operations
- Delete specific value from array
- Delete value at specific index in array
- Remove first value in array
- Remove last value in array
- Array - Notice: Undefined offset: 0
- JSON encode
- Array Functions
- array_chunk()
- array_column()
- array_combine()
- array_change_key_case()
- array_count_values()
- array_diff_assoc()
- array_diff_key()
- array_diff_uassoc()
- array_diff_ukey()
- array_diff()
- array_fill()
- array_fill_keys()
- array_flip()
- array_key_exists()
- array_keys()
- array_pop()
- array_product()
- array_push()
- array_rand()
- array_replace()
- array_sum()
- array_values()
- Create an object or instance
- Constructor
- Constants in class
- Read only properties in class
- Encapsulation
- Inheritance
- Define property and method with same name in class
- Uncaught Error: Cannot access protected property
- Nested try catch
- Multiple catch blocks
- Catch multiple exceptions in a single catch block
- Print exception message
- Throw exception
- User defined Exception
- Get current timestamp
- Get difference between two given dates
- Find number of days between two given dates
- Add specific number of days to given date
- ❯ PHP Tutorial
- ❯ PHP Operators
- ❯ Assignment
PHP Assignment Operators
In this tutorial, you shall learn about Assignment Operators in PHP, different Assignment Operators available in PHP, their symbols, and how to use them in PHP programs, with examples.
Assignment Operators
Assignment Operators are used to perform to assign a value or modified value to a variable.
Assignment Operators Table
The following table lists out all the assignment operators in PHP programming.
Operator | Symbol | Example | Description |
---|---|---|---|
Simple Assignment | = | x = 5 | Assigns value of 5 to variable x. |
Addition Assignment | += | Assigns the result of to x. | |
Subtraction Assignment | -= | Assigns the result of to x. | |
Multiplication Assignment | *= | Assigns the result of to x. | |
Division Assignment | /= | Assigns the result of to x. | |
Modulus Assignment | %= | Assigns the result of to x. |
In the following program, we will take values in variables $x and $y , and perform assignment operations on these values using PHP Assignment Operators.
PHP Program
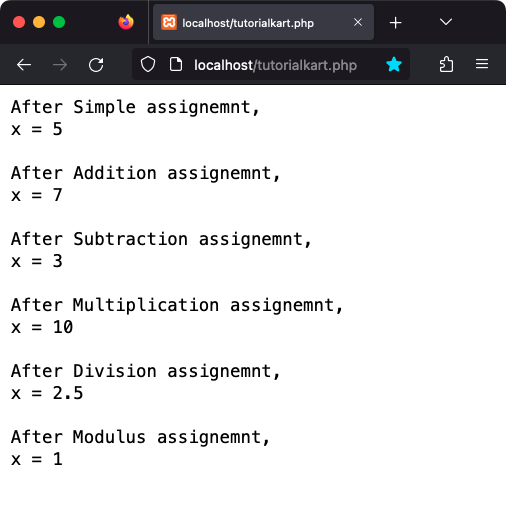
Assignment Operators Tutorials
The following tutorials cover each of the Assignment Operators in PHP in detail with examples.
- PHP Simple Assignment
- PHP Addition Assignment
- PHP Subtraction Assignment
- PHP Multiplication Assignment
- PHP Division Assignment
- PHP Modulus Assignment
In this PHP Tutorial , we learned about all the Assignment Operators in PHP programming, with examples.
Popular Courses by TutorialKart
App developement, web development, online tools.
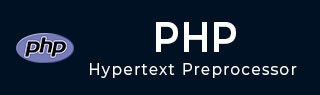
- PHP Tutorial
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook and Paypal Integration
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - Callbacks
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
PHP - Assignment Operators Examples
You can use assignment operators in PHP to assign values to variables. Assignment operators are shorthand notations to perform arithmetic or other operations while assigning a value to a variable. For instance, the "=" operator assigns the value on the right-hand side to the variable on the left-hand side.
Additionally, there are compound assignment operators like +=, -= , *=, /=, and %= which combine arithmetic operations with assignment. For example, "$x += 5" is a shorthand for "$x = $x + 5", incrementing the value of $x by 5. Assignment operators offer a concise way to update variables based on their current values.
The following table highligts the assignment operators that are supported by PHP −
Operator | Description | Example |
---|---|---|
= | Simple assignment operator. Assigns values from right side operands to left side operand | C = A + B will assign value of A + B into C |
+= | Add AND assignment operator. It adds right operand to the left operand and assign the result to left operand | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator. It subtracts right operand from the left operand and assign the result to left operand | C -= A is equivalent to C = C - A |
*= | Multiply AND assignment operator. It multiplies right operand with the left operand and assign the result to left operand | C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator. It divides left operand with the right operand and assign the result to left operand | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator. It takes modulus using two operands and assign the result to left operand | C %= A is equivalent to C = C % A |
The following example shows how you can use these assignment operators in PHP −
It will produce the following output −
- ▼PHP Operators
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Assignment Operators
- Bitwise Operators
- String Operators
- Array Operators
- Incrementing Decrementing Operators
PHP Assignment Operators
Description.
Assignment operators allow writing a value to a variable . The first operand must be a variable and the basic assignment operator is "=". The value of an assignment expression is the final value assigned to the variable. In addition to the regular assignment operator "=", several other assignment operators are composites of an operator followed by an equal sign.
Interestingly all five arithmetic operators have corresponding assignment operators, Here is the list.
The following table discussed more details of the said assignment operators.
Shorthand | Expression | Description |
---|---|---|
$a+= $b | $a = $a + $b | Adds 2 numbers and assigns the result to the first. |
$a-= $b | $a = $a -$b | Subtracts 2 numbers and assigns the result to the first. |
$a*= $b | $a = $a*$b | Multiplies 2 numbers and assigns the result to the first. |
$a/= $b | $a = $a/$b | Divides 2 numbers and assigns the result to the first. |
$a%= $b | $a = $a%$b | Computes the modulus of 2 numbers and assigns the result to the first. |
View the example in the browser
Previous: Logical Operators Next: Bitwise Operators
Follow us on Facebook and Twitter for latest update.
- Weekly Trends and Language Statistics
PHP Tutorial
Welcome to the modern PHP tutorial! This PHP tutorial helps you learn how to develop dynamic websites and web applications using PHP from scratch.
PHP is one of the most popular programming languages for web development.
PHP allows you to develop various web applications, including blogs, content management systems (CMS), and online stores.
Section 1. Getting Started with PHP
- What is PHP – quickly introduce you to PHP and its ecosystem.
- Install PHP – learn how to install a PHP development environment locally on your computer.
- Hello, World – walk you through the steps of writing and running the first PHP script.
Section 2. PHP Fundamentals
- Syntax – introduce you to the basic PHP syntax, including sensitivity, statements, whitespace, and linebreak.
- Variables – show you how to use variables to store data in PHP.
- Constants – define constants that hold a value that doesn’t change throughout the script.
- Comments – learn how to document your code effectively using comments.
- var_dump – learn how to dump the information about a variable.
Section 3. PHP Types
- Data types – give you a quick overview of PHP types, including scalar types, compound types, and special types.
- Boolean – learn how to represent a truth value using the boolean type.
- Integer – explain to you how to work with integers in PHP.
- Float – guide you on how to represent floating-point numbers.
- String – learn about various forms of strings in PHP, including single-quoted and double-quoted strings.
- Null – introduce to you the NULL type and how to check if a variable is NULL or not.
- Type Casting – learn how to cast a value of one type to another.
- Type Juggling – explain to you how the type juggling works in PHP.
Section 4. Operators
This section covers the most commonly used operators in PHP including logical and comparison operators.
- Assignment operators – learn about the most commonly used assignment operators effectively.
- Comparison operators – introduce to you the comparison operators that allow you to compare two values of the same or different types.
- Logical AND operator (&&) – show you how to use the logical AND operator to build complex logical expressions.
- Logical OR operator (||) – learn how to use the logical OR operator to form complex logical expressions.
- Logical NOT operator (!) – learn how to negate a Boolean expression.
- Operators – explain to you the available operators in PHP and help you apply them effectively.
Section 5. Control flow
- if – learn how to execute a code block based on a condition.
- if-else – show you how to run a code block if a condition is true and another code block if the condition is false .
- if-elseif – show you how to execute the corresponding code block based on multiple conditions.
- Ternary operator – guide you on using the ternary operator to make your code shorter and more readable.
- switch – learn how to use the switch statement effectively.
- for – execute a code block a fixed number of times.
- while – explain to you how to run a pretest loop.
- do-while – understand the posttest loop and learn when to use it in the script.
- break – learn how to break out of a loop.
- continue – show you how to skip the current loop iteration and start the new one from the beginning.
Section 6. Functions
- Functions – show you how to define user-defined functions.
- Function parameters – learn about function parameters, passing arguments by value and by reference.
- Default parameters – show you how to set a default value for a parameter.
- Named arguments – explain to you the named arguments that allow you to pass arguments to a function using the parameter names rather than parameter positions.
- Variable scopes – learn about the variable scopes, including global, local, static, and function parameters.
- Type hints – show you how to declare types for function parameters and return value.
- Strict typing – learn how to enable strict mode using the strict typing directive.
- Variadic functions – learn how to define function that accepts a variable number of arguments.
Section 7. Array
- Arrays – show you how to handle a list of items effectively with Array data type.
- Associative Arrays – learn how to use the associative arrays to reference elements by names rather than numbers.
- foreach – show you how to use the foreach statement to iterate over elements of an index array or an associative array.
- Multidimensional Array – guide you on how to define a multidimensional array and manipulate its elements effectively.
- array_unshift() – add one or more elements to the beginning of an array.
- array_push() – add one or more elements to the end of an array.
- array_pop() – remove an element from the end of an array and return it.
- array_shift() – remove an element from the beginning of an array and return it.
- array_keys() – get the keys of an array.
- array_key_exists() – check if a key exists in an array.
- in_array() – check if a value exists in an array.
- array_reverse() – reverse the order of elements in an array.
- array_merge() – merge multiple arrays into one.
- Spread operator – show you how to use the spread operator with an array expression.
- list – learn how to use the list construct to assign the array elements to multiple variables in a single operation.
- Array destructuring – show you how to unpack array elements to multiple variables.
Section 8. Sorting Arrays
This section introduces to you various helpful functions to sort elements of an array.
- sort – sort values of an array in the ascending order (or use the rsort() function to sort the values of an array in the descending order).
- ksort() – sort array keys of an associative array.
- usort() – sort an array with a user-defined function.
- asort() – sort an associative array and maintain the index association.
- uasort() – sort an associative array with a user-defined comparison function and maintains the index association.
- uksort() – sort the keys of an array with a user-defined comparison function.
Section 9. Advanced Functions
- Anonymous functions – learn how to use anonymous functions effectively.
- Arrow functions – show you how to use the arrow functions for writing short anonymous functions.
- Variable functions – guide you on how to call a function dynamically via a variable.
Section 10. Variable constructs
- isset – return true if a variable is set and not null.
- empty – returns true if a variable does not exist or is false.
- is_null – returns true if a variable does not exist or is null.
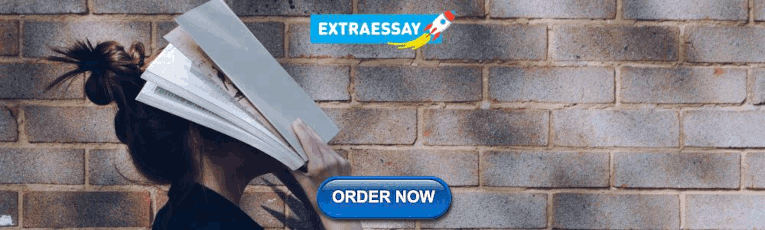
Section 11. Advanced Array Operations
- Map – map array elements using the array_map() function.
- Filter – filter the elements of an array by a callback using the array_filter() function.
- Reduce – reduce an array to a single value by a callback function using the array_reduce() funtion.
Section 12. Organizing PHP files
- Include – show you how to use the include construct to make PHP script files more organized.
- include_once – show you how to include a file once using the include_once construct.
- require & require_once – learn how to include files in a more robust way to a file with the require and require_once constructs.
- Using __DIR__ with a file include – show you how to use the __DIR__ magic constant when including a file.
- Variable variables – learn how to pass data to the included script using variable variables.
Section 13. State Management
- Cookie – learn how to store a piece of data on web browsers.
- Session – explain to you how sessions work.
Section 14. Processing Forms
- PHP form – show you how to process HTML forms.
- filter_has_var() function – check if a variable of a type exists.
- filter_var() function – sanitize and validate a variable using a filter.
- filter_input() function – sanitize and validate a variable of a type.
- Form validation – walk you through the step of validating a form in PHP.
- Checkbox – learn how to process checkboxes.
- Multiple checkboxes – show you how to handle a form that has multiple checkboxes with the same name.
- Radio button – learn how to handle radio buttons and radio groups.
- Select – show you how to handle the select element.
- CSRF – learn about CSRF attacks and how to prevent them by using a one-time token in PHP.
- Flash messages – show you how to implement session-based flash messages for creating and displaying messages across pages.
- Post-Redirect-Get (PRG) – learn how to use the PRG technique to prevent the double submit problem.
- File Upload – guide you on how to upload a file to the server securely.
- Upload multiple files – show you how to upload multiple files to the server securely.
- Contact form – show you how to create a contact form that sends email and prevent spam.
- Validation – learn how to build a reusable validate() function to validate inputs.
- Sanitize input – learn how to implement a reusable sanitize() function to sanitize inputs.
- Filter input – define a filter() helper furnction that both santizes and validates data.
- password_hash() – show you how to create a hash from a plain text password using a secure hashing algorithm.
- password_verify() – guide you on how to match a password with a hash.
Section 15. Login System
This section shows you how to use the learned skills and knowledge to build a simple PHP login system.
- Registration – show you how to build a account registration form.
- Login – learn how to create a login form.
- Email verification – add the email verification feature when users register for new accounts.
- Remember me – enhance the login form by adding a remember me checkbox to save the login even after closing the web browser.
Section 16. Working with Files
- Open a file – learn how to open a file for reading and/or writing using the fopen() function.
- Check a File Exists – show you how to check if a file exists using the file_exists(), is_readable(), and is_writable() functions.
- Read a file – show you how to read the contents of a file into a string or read a file line by line into a string.
- Read a file into a string – guide you on using the file_get_contents() function to read a file into a string.
- Read a file into an array – show you how to read the contents of a file into an array, each line of the file is an array element.
- Download a file – show you how to download a file from the webserver.
- Copy a file – show you how to copy a file to a new one.
- Delete a file – learn how to delete a file.
- Rename a file – guide you on renaming a file to a new one and optionally move it to another directory.
- Work with CSV Files – learn how to create and read CSV files in PHP.
- Get the size of a file – show how to get the size of a file in bytes using the filesize() function.
- File permissions – learn about the file permission and how to change it using the chmod() function.
Section 17. Working with Directories
- Manage directory – show you how to manage directories in PHP.
- glob – get files/directories in a directory that match a pattern.
- dirname – get the directory name of a path.
- basename – get the trailing name component of a path.
- pathinfo – return the components of a file path including directory name, trailing name component, filename, and file extension.
Section 18. String operations
- strlen – return the length of a string in bytes.
- substr – search for a substring in a string.
- strpos – locate the first occurrence of a substring in a string.
- str_replace – replace all occurrences of a substring with a new one in a string.
- implode – join an array of strings into a string using a separator.
- explode – split a string by a separator into an array of strings.
- trim – remove the whitespace characters or other characters from both ends of a string.
- ltrim – remove the whitespace characters or other characters from the beginning of a string.
- rtrim – remove the whitespace characters or other characters from the end of a string.
- htmlspecialchars() – show you how to escape special characters in a string.
- str_contains() – return true if a string contains with a substring.
- str_starts_with() – return true if a string starts with a substring.
- str_ends_with() – return true if a string ends with a substring.
- strtolower() – accept a string and return a new string with all alphabetic characters converted to lowercase.
- strtoupper() – accept a string and return a new string with all alphabetic characters converted to uppercase.
- ucfirst() – return a new string with the first alphabetic character converted to uppercase.
- ucwords() – return a new string with the first alphabetic character of each word converted to uppercase.
Section 19. Regular Expressions
- Regular expressions – introduce you to the regular expressions and show you how to use the preg_match(), preg_match_all(), and preg_replace() functions.
- Character Classes – match a character in a character set ( \d , \w , \s )
- Anchors – match at the beginning ( ^ ) and/or end ( $ ) of a string or line.
- Word Boundary – match the whole word using the word boundary ( \b ).
- Sets & Ranges – match a character in a set ( [abc] ) or range of characters ( [a-z] ).
- Quantifiers – match a number of instances of a character or character class using quantifiers ( \d{2,4} , \w+ …).
- Greedy Quantifiers – learn how the greedy quantifiers work.
- Non-greedy (or lazy) Quantifiers – show you how to turn a greedy quantifier into a non-greedy quantifiers and explain how the non-greedy quantifiers work.
- Capturing groups – include a part of a match in the matches array and assign it a name using a named group.
- Backreferences – learn about the regex backreferences.
- Alternation – show you how to use the alternation, which is simply an OR operator in regular expressions.
- Lookahead – learn how to match A only if followed by B.
- Lookbehind – learn how to match A only if there’s is B before it.
- preg_match() – search for a match in a string using a regular expression.
- preg_match_all() – search for all matches in a string using a regular expression.
- preg_replace() – search and replace strings using a regular expression.
Section 20. PHP Date & Time
- Time – learn how to work with time.
- Date – show you how to use the date() function to format a timestamp.
Learn PHP Variables
Print Cheatsheet
Parsing Variables within PHP Strings
In PHP, variables can be parsed within strings specified with double quotes ( " ).
This means that within the string, the computer will replace an occurence of a variable with that variable’s value.
When additional valid identifier characters (ie. characters that could be included in a variable name) are intended to appear adjacent to the variable’s value, the variable name can be wrapped in curly braces {} , thus avoiding confusion as to the variable’s name.
Reassignment of PHP Variables
In PHP, variables are assigned values with the assignment operator ( = ). The same variable can later be reassigned a new value using the same operator.
This process is known as reassignment .
Concatenating Strings in PHP
In PHP, if you want to join two strings together, you need to use the . operator.
This process is called concatenation . Put the . operator between the two strings in order to join them.
Note that strings are joined as-is, without inserting a whitespace character. So if you need to put spaces, you need to incorporate the whitespace manually within the string.
Appending a String in PHP
In PHP, there is a shortcut for appending a new string to the end of another string. This can be easily done with the string concatenation assignment operator ( .= ).
This operator will append the value on its right to the value on its left and then reassign the result to the variable on its left.
PHP Strings
In PHP, a string is a sequence of characters surrounded by double quotation marks. It can be as long as you want and contain any letters, numbers, symbols, and spaces.
PHP copying variables
In PHP, one variable’s value can be assigned to another variable.
This creates a copy of that variable’s value and assigns the new variable name to it.
Changes to the original variable will not affect the copy and changes to the copy will not affect the original. These variables are entirely separate entities.
PHP String Escape Sequences
In PHP, sometimes special characters must be escaped in order to include them in a string. Escape sequences start with a backslash character ( \ ).
There are a variety of escape sequences that can be used to accomplish different tasks. For example, to include a new line within a string, the sequence \n can be used. To include double quotation marks, the sequence \" can be used. Similarly, to include single quotes, the sequence \' can be used.
PHP Evaluation Order during Assignment
In PHP, when an assignment takes place, operations to the right of the assignment operator ( = ) will be evaluated to a single value first. The result of these operations will then be assigned to the variable.
PHP Variables
In PHP, variables are assigned values with the assignment operator ( = ).
Variable names can contain numbers, letters, and underscores ( _ ). A sigil ( $ ) must always precede a variable name. They cannot start with a number and they cannot have spaces or any special characters.
The convention in PHP is to use snake case for variable naming; this means that lowercase words are delimited with an underscore character ( _ ). Variable names are case-sensitive.
PHP Reference Assignment Operator
In PHP, the reference assignment operator ( =& ) is used to create a new variable as an alias to an existing spot in memory.
In other words, the reference assignment operator ( =& ) creates two variable names which point to the same value. So, changes to one variable will affect the other, without having to copy the existing data.
Integer Values in PHP
PHP supports integer values for numbers.
Integers are the set of all whole numbers, their negative counterparts, and zero. In other words, an integer is a number of the set ℤ = {…, -2, -1, 0, 1, 2, …}.
Exponentiation Operator in PHP
PHP supports an arithmetic operator for exponentiation ( ** ).
This operator gives the result of raising the value on the left to the power of the value on the right.
Arithmetic Operators in PHP
PHP supports arithmetic operators for addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ).
PHP operators will return integers whenever the result of an operation evaluates to a whole number. If the result evaluates to a fraction or decimal, then it will return a floating point number .
The Modulo Operator
PHP supports a modulo operator ( % ). The modulo operator returns the remainder of the left operand divided by the right operand. Operands of a modulo operation are converted to integers prior to performing the operation. The operation returns an integer with the same sign as the dividend.
Floating Point Numbers in PHP
PHP supports floating-point (decimal) numbers. They can be used to represent fractional quantities as well as precise measurements. Some examples of floating point numbers are 1.5 , 4.231 , 2.0 , etc.
- Language Reference
Logical Operators
Example | Name | Result |
---|---|---|
$a and $b | And | if both and are . |
$a or $b | Or | if either or is . |
$a xor $b | Xor | if either or is , but not both. |
! $a | Not | if is not . |
$a && $b | And | if both and are . |
$a || $b | Or | if either or is . |
The reason for the two different variations of "and" and "or" operators is that they operate at different precedences. (See Operator Precedence .)
Example #1 Logical operators illustrated
The above example will output something similar to:
Improve This Page
User contributed notes 14 notes.

- PHP Tutorial
- PHP Exercises
- PHP Calendar
- PHP Filesystem
- PHP Programs
- PHP Array Programs
- PHP String Programs
- PHP Interview Questions
- PHP IntlChar
- PHP Image Processing
- PHP Formatter
- Web Technology
PHP Operators
In this article, we will see how to use the operators in PHP, & various available operators along with understanding their implementation through the examples.
Operators are used to performing operations on some values. In other words, we can describe operators as something that takes some values, performs some operation on them, and gives a result. From example, “1 + 2 = 3” in this expression ‘+’ is an operator. It takes two values 1 and 2, performs an addition operation on them to give 3.
Just like any other programming language, PHP also supports various types of operations like arithmetic operations(addition, subtraction, etc), logical operations(AND, OR etc), Increment/Decrement Operations, etc. Thus, PHP provides us with many operators to perform such operations on various operands or variables, or values. These operators are nothing but symbols needed to perform operations of various types. Given below are the various groups of operators:
- Arithmetic Operators
- Logical or Relational Operators
- Comparison Operators
- Conditional or Ternary Operators
- Assignment Operators
- Spaceship Operators (Introduced in PHP 7)
- Array Operators
- Increment/Decrement Operators
- String Operators
Let us now learn about each of these operators in detail.
Arithmetic Operators:
The arithmetic operators are used to perform simple mathematical operations like addition, subtraction, multiplication, etc. Below is the list of arithmetic operators along with their syntax and operations in PHP.
+ | Addition | $x + $y | Sum the operands |
– | Subtraction | $x – $y | Differences the operands |
* | Multiplication | $x * $y | Product of the operands |
/ | Division | $x / $y | The quotient of the operands |
** | Exponentiation | $x ** $y | $x raised to the power $y |
% | Modulus | $x % $y | The remainder of the operands |
Note : The exponentiation has been introduced in PHP 5.6.
Example : This example explains the arithmetic operator in PHP.
Logical or Relational Operators:
These are basically used to operate with conditional statements and expressions. Conditional statements are based on conditions. Also, a condition can either be met or cannot be met so the result of a conditional statement can either be true or false. Here are the logical operators along with their syntax and operations in PHP.
and | Logical AND | $x and $y | True if both the operands are true else false |
or | Logical OR | $x or $y | True if either of the operands is true else false |
xor | Logical XOR | $x xor $y | True if either of the operands is true and false if both are true |
&& | Logical AND | $x && $y | True if both the operands are true else false |
|| | Logical OR | $x || $y | True if either of the operands is true else false |
! | Logical NOT | !$x | True if $x is false |
Example : This example describes the logical & relational operator in PHP.
Comparison Operators: These operators are used to compare two elements and outputs the result in boolean form. Here are the comparison operators along with their syntax and operations in PHP.
Operator | Name | Syntax | Operation |
---|---|---|---|
== | Equal To | $x == $y | Returns True if both the operands are equal |
!= | Not Equal To | $x != $y | Returns True if both the operands are not equal |
<> | Not Equal To | $x <> $y | Returns True if both the operands are unequal |
=== | Identical | $x === $y | Returns True if both the operands are equal and are of the same type |
!== | Not Identical | $x == $y | Returns True if both the operands are unequal and are of different types |
< | Less Than | $x < $y | Returns True if $x is less than $y |
> | Greater Than | $x > $y | Returns True if $x is greater than $y |
<= | Less Than or Equal To | $x <= $y | Returns True if $x is less than or equal to $y |
>= | Greater Than or Equal To | $x >= $y | Returns True if $x is greater than or equal to $y |
Example : This example describes the comparison operator in PHP.
Conditional or Ternary Operators :
These operators are used to compare two values and take either of the results simultaneously, depending on whether the outcome is TRUE or FALSE. These are also used as a shorthand notation for if…else statement that we will read in the article on decision making.
Here, the condition will either evaluate as true or false. If the condition evaluates to True, then value1 will be assigned to the variable $var otherwise value2 will be assigned to it.
?: | Ternary | If the condition is true? then $x : or else $y. This means that if the condition is true then the left result of the colon is accepted otherwise the result is on right. |
Example : This example describes the Conditional or Ternary operators in PHP.
Assignment Operators: These operators are used to assign values to different variables, with or without mid-operations. Here are the assignment operators along with their syntax and operations, that PHP provides for the operations.
= | Assign | $x = $y | Operand on the left obtains the value of the operand on the right |
+= | Add then Assign | $x += $y | Simple Addition same as $x = $x + $y |
-= | Subtract then Assign | $x -= $y | Simple subtraction same as $x = $x – $y |
*= | Multiply then Assign | $x *= $y | Simple product same as $x = $x * $y |
/= | Divide then Assign (quotient) | $x /= $y | Simple division same as $x = $x / $y |
%= | Divide then Assign (remainder) | $x %= $y | Simple division same as $x = $x % $y |
Example : This example describes the assignment operator in PHP.
Array Operators: These operators are used in the case of arrays. Here are the array operators along with their syntax and operations, that PHP provides for the array operation.
+ | Union | $x + $y | Union of both i.e., $x and $y |
== | Equality | $x == $y | Returns true if both has same key-value pair |
!= | Inequality | $x != $y | Returns True if both are unequal |
=== | Identity | $x === $y | Returns True if both have the same key-value pair in the same order and of the same type |
!== | Non-Identity | $x !== $y | Returns True if both are not identical to each other |
<> | Inequality | $x <> $y | Returns True if both are unequal |
Example : This example describes the array operation in PHP.
Increment/Decrement Operators: These are called the unary operators as they work on single operands. These are used to increment or decrement values.
++ | Pre-Increment | ++$x | First increments $x by one, then return $x |
— | Pre-Decrement | –$x | First decrements $x by one, then return $x |
++ | Post-Increment | $x++ | First returns $x, then increment it by one |
— | Post-Decrement | $x– | First returns $x, then decrement it by one |
Example : This example describes the Increment/Decrement operators in PHP.
String Operators: This operator is used for the concatenation of 2 or more strings using the concatenation operator (‘.’). We can also use the concatenating assignment operator (‘.=’) to append the argument on the right side to the argument on the left side.
. | Concatenation | $x.$y | Concatenated $x and $y |
.= | Concatenation and assignment | $x.=$y | First concatenates then assigns, same as $x = $x.$y |
Example : This example describes the string operator in PHP.
Spaceship Operators :
PHP 7 has introduced a new kind of operator called spaceship operator. The spaceship operator or combined comparison operator is denoted by “<=>“. These operators are used to compare values but instead of returning the boolean results, it returns integer values. If both the operands are equal, it returns 0. If the right operand is greater, it returns -1. If the left operand is greater, it returns 1. The following table shows how it works in detail:
$x < $y | $x <=> $y | Identical to -1 (right is greater) |
$x > $y | $x <=> $y | Identical to 1 (left is greater) |
$x <= $y | $x <=> $y | Identical to -1 (right is greater) or identical to 0 (if both are equal) |
$x >= $y | $x <=> $y | Identical to 1 (if left is greater) or identical to 0 (if both are equal) |
$x == $y | $x <=> $y | Identical to 0 (both are equal) |
$x != $y | $x <=> $y | Not Identical to 0 |
Example : This example illustrates the use of the spaceship operator in PHP.
Please Login to comment...
Similar reads.
- Web Technologies
- PHP-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Prospective Students
- Current Students
- Faculty & Staff
Middlesex Campus Library
Middlesex distance learning blackboard tutorials.
- Blackboard Windows
- Syllabus & Files
- Discussions
- Mail -Messages
- Announcements & Calendar
- Exams/Tests
Submit an Assignment
View an assignment grade and feedback.
- Collaborate
- Distance Learning
Your professor may ask you to submit a class assignment (e.g. research paper, project, etc) using an Assignment dropbox . Assignment dropboxes could be placed on a page named Assignments from the Course Menu or in a week or a unit folder.
In this tutorial, the assignment dropbox for the case study is placed in the Unit 2 folder on Course Content page. 1. Click on Course Content on the Course Menu . Click on Unit 2 . 2. You will see the assignment dropbox with the instruction about the assignment. Click on the Unit 2 Assignment link. 3. In most cases, your instructor asks you to submit the assignment as an attached file, click on Upload Files and then click on Browse Local Files. Locate your assignment file saved on your computer and double-click on the file. You will see the file listed underneath Attach Files . 4. For submitting multiple files, click on Browse Local Files again and select another file . ( Use "Do Not Attach" to remove a file mistakenly attached.) 5 . Before you proceed, check the attached file (or files), making sure that all files are attached. You may write a brief message under ADD COMMENTS box. Click on Submit . Then you see the assignment file, submission date, and grading status under Review Submission History . Click on OK to confirm. 6. After you have confirmed your submission, you will receive an email in your college email account. You are recommended keep this email for later reference. 7. If your professor sets up one attempt on the assignment dropbox, after you have submitted an assignment file, you will not be able to resubmit the file again. If you submit a wrong file but you are unable to resubmit, you should contact your professor to have your submission reset.
After your professor has graded your assignment, you can view your assignment grade and your professor's feedback via the assignment link or My Grades .
1. View the assignment grade and feedback directly from the Assignment link. When the assignment is still within its availability, after your professor has graded your work, you can view your grade and your professor's feedback directly from the Assignment link.
In this example, the assignment is located in the Unit 2 folder under Course Content . (1) Click on the Course Content on the Course Menu and click on the Unit 2 . Click on the assignment name . You will see a grade and feedback from your professor. (2) You may also see the feedback from your professor directly on the file you have submitted.
2. View the assignment grade and feedback in My Grades.
You can go to My Grades to view your assignment grade and your professor's feedback, especially when an assignment has passed due and the assignment link is not available.
(1) Click on My Grades link on the Course Menu .
(2) In the row of the assignment, you will see your grade. Click on the feedback (bubble) icon next to the grade value, you will see your professor's feedback. When you click on the assignment name, you may see your professor's feedback right on the assignment file.
- << Previous: Exams/Tests
- Next: Collaborate >>
- Last Updated: Aug 12, 2024 3:36 PM
- URL: https://library.ctstate.edu/BbTutorials
PHP Tutorial
Php advanced, mysql database, php examples, php reference, php variables.
Variables are "containers" for storing information.
Creating (Declaring) PHP Variables
In PHP, a variable starts with the $ sign, followed by the name of the variable:
In the example above, the variable $x will hold the value 5 , and the variable $y will hold the value "John" .
Note: When you assign a text value to a variable, put quotes around the value.
Note: Unlike other programming languages, PHP has no command for declaring a variable. It is created the moment you first assign a value to it.
Think of variables as containers for storing data.
A variable can have a short name (like $x and $y ) or a more descriptive name ( $age , $carname , $total_volume ).
Rules for PHP variables:
- A variable starts with the $ sign, followed by the name of the variable
- A variable name must start with a letter or the underscore character
- A variable name cannot start with a number
- A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
- Variable names are case-sensitive ( $age and $AGE are two different variables)
Remember that PHP variable names are case-sensitive!
Advertisement
Output Variables
The PHP echo statement is often used to output data to the screen.
The following example will show how to output text and a variable:
The following example will produce the same output as the example above:
The following example will output the sum of two variables:
Note: You will learn more about the echo statement and how to output data to the screen in the PHP Echo/Print chapter .
PHP is a Loosely Typed Language
In the example above, notice that we did not have to tell PHP which data type the variable is.
PHP automatically associates a data type to the variable, depending on its value. Since the data types are not set in a strict sense, you can do things like adding a string to an integer without causing an error.
In PHP 7, type declarations were added. This gives an option to specify the data type expected when declaring a function, and by enabling the strict requirement, it will throw a "Fatal Error" on a type mismatch.
You will learn more about strict and non-strict requirements, and data type declarations in the PHP Functions chapter.
Variable Types
PHP has no command for declaring a variable, and the data type depends on the value of the variable.
PHP supports the following data types:
- Float (floating point numbers - also called double)
Get the Type
To get the data type of a variable, use the var_dump() function.
The var_dump() function returns the data type and the value:
See what var_dump() returns for other data types:
Assign String to a Variable
Assigning a string to a variable is done with the variable name followed by an equal sign and the string:
String variables can be declared either by using double or single quotes, but you should be aware of the differences. Learn more about the differences in the PHP Strings chapter .
Assign Multiple Values
You can assign the same value to multiple variables in one line:
All three variables get the value "Fruit":

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
assigning values to a variable in if condition in php
Can anybody explain how assigning values to variables work in php. I have the following code which does not work as expected (by me).
If I use this code account is 1, just ONE. How come??? getClientAccount returns string (username) or false if not user found. When user is found that 1 is returned. If I move the assignment above if statement, it works fine. I have similar problem when assining array returned by function to variable in if statement.
Alright, guys I think I discovered something, at least something for all of us here. Look at this code: http://codepad.org/QWjwl3vQ I think you will understand what is happening. Just try test1() and test2()
- if-statement
- variable-assignment
- 1 Why semi colon in if statement condition ? – Rikesh Commented Nov 21, 2013 at 6:31
- edited. removed that semicolon. It was not in my code actually – Jamol Commented Nov 21, 2013 at 6:43
- If possible show your func. getClientAccount , so that one can have a better idea. – Rikesh Commented Nov 21, 2013 at 6:50
- Pay attention to my words: If I move the assignment above if statement, it works fine, as expected – Jamol Commented Nov 21, 2013 at 7:34
- Please have a look on edit. – Jamol Commented Nov 21, 2013 at 7:43
6 Answers 6
After edit & checking your code it seems that boolean value is getting over write on the actual value. To get rid of this thing you need round braces on each condition for proper assignment called Operator Precedence .
Check your code that I have edited to make work as you expected.

- You made it. Thanks a lot. But I didn`t get why it was not working without extra braces – Jamol Commented Nov 21, 2013 at 7:59
- Your welcome !! Check the operator precedence reference I added in my answer for details. – Rikesh Commented Nov 21, 2013 at 8:00
The first block of code is syntactically wrong.

That's simply not a real question and there is no point in trying to answer it directly, especially if you aren't familiar with PHP syntax.
It's the OP's premises have to be questioned, not the code he's posted.
This code will plainly output the result of getClientAccount method (unless it's 0 or empty array).
So, the OP have to check what "was in the code actually" once more or verify the actual result of getClientAccount function.

- This your new question is totally different from one you asked. You have to make your mind before asking a question. – Your Common Sense Commented Nov 21, 2013 at 8:22
- It is exactly same thing. Read it carefully! – Jamol Commented Nov 21, 2013 at 9:52
- if you still think there is no difference, then you didn't get the answer you accepted – Your Common Sense Commented Nov 21, 2013 at 10:03
remove semicolon from if condition like following
and in your case php will check the value of $account variable after getting the returned value from the getClientAccount() function. if $account value ultimately mean true then it will execute your echo statement.
if you have any confusion about php boolean values then you can try it another way:

There are few syntax error. You need to remove the semi colon as mentioned already and you need to change to:
To check for a condition, at the moment you are trying to assign $account to $this->getClientAccount($request) result in a if statement. If statements are used to check conditions not to assign values.
Also $account already has to have a value otherwise your condition will always remain false, if the function returns a value.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged php if-statement variable-assignment or ask your own question .
- The Overflow Blog
- How we’re making Stack Overflow more accessible
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Introducing an accessibility dashboard and some upcoming changes to display...
- Tag hover experiment wrap-up and next steps
Hot Network Questions
- Is the license name and the URL to full license text sufficient for distributed software?
- Tensor algebra and universal enveloping algebra
- A 111 years old mosaic
- Communicate the intention to resign
- Can an elf and a firbolg breed?
- A Faster Method for Converting Meters to Degrees
- What is the Mac OS equilivent of linux's `hostname -I`?
- Zsh: enable extended_glob inline in a filename generation glob
- Is the word ekklesia ever used to describe unbelievers?
- Automatically closing a water valve after a few minutes
- Proving an inequality involving a definite integral.
- I'm 78 and 57 years ago I was a 1 dan. Started karate at 5. I quit karate at 21 for reasons. Want to start again. Do not remember much. What do I do.?
- Direct limits in homotopy category
- Why do instructions for various goods sold in EU nowadays lack pages in English?
- Do comets ever run out of water?
- Do radios need a minimum range to function properly?
- Flow Decision Passing when it should fail
- How to make a case based on factual evidence that my colleague's writing style for submitted manuscripts has got to be overhauled?
- What does "contradictions trivialize truth" mean?
- Special generating sets of the additive group of rational numbers
- What tool has a ring on the end of a threaded handle shaft?
- To "Add Connector" or not to "Add Connector", that is a question
- Variant of global failure of GCH
- MPs assuming office on the day of the election
Advertisement
Supported by
As Republicans Attack Harris on Immigration, Here’s What Her Record Shows
Republicans blame Vice President Kamala Harris for the surge of migrants into the United States over the past several years. But a review of her involvement shows a more nuanced record.
- Share full article

By Zolan Kanno-Youngs and Jazmine Ulloa
Reporting from Washington
As they seek effective attack lines against Vice President Kamala Harris, Republicans are focusing on her role in the Biden administration’s border and immigration policies, seeking to blame her for the surge of migrants into the United States over the past several years.
A review of her involvement in the issue shows a more nuanced record.
President Biden did not assign her the job title of “border czar” or the responsibility of overseeing the enforcement policies at the U.S.-Mexico border, as the Trump campaign suggested on Tuesday in its first ad against her. But she did have a prominent role in trying to ensure that a record surge of global migration did not become worse.
After the number of migrants crossing the southern border hit record levels at times during the administration’s first three years, crossings have now dropped to their lowest levels since Mr. Biden and Ms. Harris took office.
Her early efforts at handling her role and the administration’s policies were widely panned, even by some Democrats, as clumsy and counterproductive, especially in displaying defensiveness over why she had not visited the border. Some of her allies felt she had been handed a no-win portfolio.
Early in the administration, Ms. Harris was given a role that came to be defined as a combination of chief fund-raiser and conduit between business leaders and the economies of Guatemala, Honduras and El Salvador. Her attempt to convince companies across the world to invest in Central America and create jobs for would-be migrants had some success, according to immigration experts and current and former government officials.
But those successes only underlined the scale of the gulf in economic opportunity between the United States and Central America, and how policies to narrow that gulf could take years or even generations to show results.
We are having trouble retrieving the article content.
Please enable JavaScript in your browser settings.
Thank you for your patience while we verify access. If you are in Reader mode please exit and log into your Times account, or subscribe for all of The Times.
Thank you for your patience while we verify access.
Already a subscriber? Log in .
Want all of The Times? Subscribe .
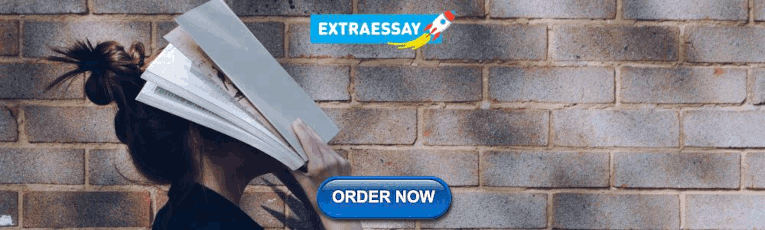
IMAGES
COMMENTS
PHP is a popular general-purpose scripting language that powers everything from your blog to the most popular websites in the world. Downloads; ... An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference. Objects may be explicitly copied via the clone keyword.
Use PHP assignment operator ( =) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
PHP All Exercises & Assignments. Practice your PHP skills using PHP Exercises & Assignments. Tutorials Class provides you exercises on PHP basics, variables, operators, loops, forms, and database. Once you learn PHP, it is important to practice to understand PHP concepts. This will also help you with preparing for PHP Interview Questions.
Assignment Operators. The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". ... An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference. Objects may be explicitly copied via the clone keyword. Assignment by Reference.
PHP Assignment Operators. The PHP assignment operators are used with numeric values to write a value to a variable. The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
Weekly Trends and Language Statistics. PHP Exercises, Practice, Solution: PHP (recursive acronym for PHP: Hypertext Preprocessor) is a widely-used open source general-purpose scripting language that is especially suited for web development and can be embedded into HTML.
PHP assignment operators applied to assign the result of an expression to a variable. = is a fundamental assignment operator in PHP. It means that the left operand gets set to the value of the assignment expression on the right. Operator. Description.
In this tutorial, you shall learn about Assignment Operators in PHP, different Assignment Operators available in PHP, their symbols, and how to use them in PHP programs, with examples. Assignment Operators. Assignment Operators are used to perform to assign a value or modified value to a variable. Assignment Operators Table
PHP - Assignment Operators Examples - You can use assignment operators in PHP to assign values to variables. Assignment operators are shorthand notations to perform arithmetic or other operations while assigning a value to a variable. For instance, the = operator assigns the value on the right-hand side to the variable on the left-han
PHP Assignment Operators Last update on August 19 2022 21:50:39 (UTC/GMT +8 hours) Description. Assignment operators allow writing a value to a variable. The first operand must be a variable and the basic assignment operator is "=". The value of an assignment expression is the final value assigned to the variable.
An operator is something that takes one or more values (or expressions, in programming jargon) and yields another value (so that the construction itself becomes an expression). Operators can be grouped according to the number of values they take. Unary operators take only one value, for example ! (the logical not operator) or ++ (the increment ...
Section 4. Operators. This section covers the most commonly used operators in PHP including logical and comparison operators. Assignment operators - learn about the most commonly used assignment operators effectively.; Comparison operators - introduce to you the comparison operators that allow you to compare two values of the same or different types. ...
There are two string operators. The first is the concatenation operator ('.'), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator (' .= '), which appends the argument on the right side to the argument on the left side. Please read Assignment Operators for more information.
It's called assignment by reference, which, to quote the manual, "means that both variables end up pointing at the same data, and nothing is copied anywhere". The only thing that is deprecated with =& is "assigning the result of new by reference" in PHP 5 , which might be the source of any confusion.
In PHP, the reference assignment operator (=&) is used to create a new variable as an alias to an existing spot in memory. In other words, the reference assignment operator (=&) creates two variable names which point to the same value. So, changes to one variable will affect the other, without having to copy the existing data.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
PHP is a popular general-purpose scripting language that powers everything from your blog to the most popular websites in the world. Downloads; Documentation; Get Involved; Help; Getting Started ... To assign default value in variable assignation, the simpliest solution to me is: <?php
In PHP, the '=' operator is used for assignment, while the '==' operator is used for loose equality comparison, meaning it checks if two values are equal without considering their data types. On the other hand, the '===' operator is used for strict equality comparison, meaning it checks if two values are equal and of the same data type. = OperatorT
Video - Submit an Assignment Your professor may ask you to submit a class assignment (e.g. research paper, project, etc) using an Assignment dropbox.Assignment dropboxes could be placed on a page named Assignments from the Course Menu or in a week or a unit folder.. In this tutorial, the assignment dropbox for the case study is placed in the Unit 2 folder on Course Content page.
It may not be immediately obvious, but the second assignment would only occur if the first returned >0, and if func(y) had other side effects that you were expecting, they would not happen either. In short, if you know what you are doing and understand the side effects, then there is nothing wrong with it.
We, the APA Style team, are not robots. We can all pass a CAPTCHA test, and we know our roles in a Turing test.And, like so many nonrobot human beings this year, we've spent a fair amount of time reading, learning, and thinking about issues related to large language models, artificial intelligence (AI), AI-generated text, and specifically ChatGPT.
Rules for PHP variables: A variable starts with the $ sign, followed by the name of the variable. A variable name must start with a letter or the underscore character. A variable name cannot start with a number. A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
Houston ISD parents hoping to send their children to school on buses scrambled to find alternatives for the first day of school on Monday, with a lack of communication on route assignments or bus ...
That's simply not a real question and there is no point in trying to answer it directly, especially if you aren't familiar with PHP syntax.. It's the OP's premises have to be questioned, not the code he's posted.. This code will plainly output the result of getClientAccount method (unless it's 0 or empty array).. So, the OP have to check what "was in the code actually" once more or verify the ...
As they seek effective attack lines against Vice President Kamala Harris, Republicans are focusing on her role in the Biden administration's border and immigration policies, seeking to blame her ...